Cango User Guide
Cango
Cango is a graphics library for the HTML5 canvas element. Cango supplies a canvas with a world coordinate system optionally with independent X and Y scaling. Both the standard Right Handed Cartesian coordinates and SVG (left handed Cartesian) coordinates are supported. Cango supports drawing Path, Shape and ClipMask objects the outlines of which are defined using the compact SVG path syntax. Cango also draws Img and Text objects. Multiple objects can be grouped as the children of a Group object so that they can be handled as a single entity. Groups can have both objects and more groups as children forming a tree structure of arbitrary depth.
Animation and drag-n-drop movements are created by applying matrix transforms; translate, rotate etc. Transforms applied to a Group are inherited by the group's children.
The latest version of Cango is 12v05, it is open source and the source code may be downloaded at Cango-12v05.js and the minified version at Cango-12v05-min.js.
Cango constructor
Syntax:
var cgo = new Cango(canvasID);
Description:
To start drawing an instance of the Cango graphics object must be created. This requires a canvas element to exist in the web page and for it to have a unique ID. Pass the canvas ID to the Cango constructor and the Cango graphics object is returned.
Parameters:
canvasID:String - The 'id' property of the canvas object on which the Cango library will draw.
Returns:
Cango object - An instance of the Cango graphics context which has all the following properties and methods.
Cango properties
Property | Type | Description |
---|---|---|
ctx | CanvasRenderingContext2D | The raw canvas drawing context. This is available to access the more esoteric canvas capabilities. |
xscl | Number | The X axis scaling factor. World coordinate X axis values are multiplied by cgo.xscl to convert to pixels. |
yscl | Number | The Y axis scaling factor. World coordinate Y axis values are multiplied by cgo.yscl to convert to pixels. cgo.yscl will always be a negative value for RHC coordinate systems and positive for SVG coordinate systems. |
heightPW | Number | The canvas height expressed as a percentage of the canvas width. cgo.heightPW is often useful in setting gridboxPadding. |
widthPW | Number | The canvas width expressed as a percentage of the canvas width, so always equal to 100. (Provided for completeness with the more useful heightPW). |
aRatio | Number | Aspect ratio of the canvas element, the canvas width divided by canvas height. If the canvas is 300px wide and 200px high, cgo.aRatio = 1.5. |
rawWidth | Number | The canvas width in pixels. |
rawHeight | Number | The canvas height in pixels. |
Cango Methods
Setting up world coordinates
A Cango graphics context refers all its drawing dimensions to a world coordinate grid which can be either the standard Right Handed Cartesian coordinates (RHC) or the Left Handed Cartesian coordinates as used in the SVG (Scalar Vector Graphics) system. World coordinates can have different X and Y scaling which greatly simplifies the plotting of numeric data.
A RHC coordinate system is established by calling Cango's setWorldCoordsRHC method.
cgo.setWorldCoordsRHC([gbLowerLeftX, gbLowerLeftY, gbWidth [, gbHeight]]);
This defines the X, Y coordinates of the LOWER LEFT corner of the gridbox which is the full canvas by default, and the width and height of the gridbox in world coordinate units. X and Y dimensions can be in different units. If square pixels are desired, omit the 'gridboxHeight' argument and the Y scale factor will be set equal to the X scale factor. X coordinate values increase to the RIGHT and Y coordinates increase UP the canvas as shown on the left of Fig 1.
An SVG or left handed Cartesian coordinate system is established by calling the Cango.setWorldCoordsSVG method.
cgo.setWorldCoordsSVG([gbUpperLeftX, gbUpperLeftY, gbWidth [, gbHeight]]);
This defines the X, Y coordinates of the UPPER LEFT corner of the gridbox, and the width and height of the gridbox in world coordinate units. X and Y dimensions can be in different units. If square pixels are desired, omit the 'gbHeight' argument and the Y scale factor will be set equal to the X scale factor. X coordinate values increase to the RIGHT and Y coordinates increase DOWN the canvas as shown on the right of Fig 1.
Setting the gridbox
Every Cango context has its own gridbox, multiple Cango contexts on the same canvas all have independent world coordinates set with reference to its gridbox. Every new Cango instance has its gridbox set to the full canvas by default. Cango provides control of the gridbox dimensions by defining a padding width from each edge of the canvas. Padding the gridbox is particularly useful when plotting graphs on the canvas element since the padding around the gridbox gives room for scales and annotation of the graph axes.
cgo.gridboxPadding(left, bottom, right, top);
The gridbox padding width is expressed as a percentage of the canvas width. Fig 2 shows examples of gridboxPadding and the way setWorldCoordsRHC and setWorldCoordsSVG use the gridbox to define their coordinates. Axes have been drawn to demonstrate the different scaling. The methods called were:
cgo.gridboxPadding(10, 10, 5, 5); cgo.setWorldCoordsRHC(0, 0, 250, 3); cgo.drawAxes();
cgo.gridboxPadding(10, 5, 5, 10); cgo.setWorldCoordsSVG(0, 0, 250, 3); cgo.drawAxes();
Syntax:
cgo.gridboxPadding([left[, bottom[, right[, top]]]]);
Description:
Every Cango context has its own gridbox which provides the reference point for the world coordinate origin and the gridbox width and height provide a reference distance for setting the world coordinate scaling in the X and Y directions. Gridbox position and dimensions are controlled by setting the padding from each side to the corresponding canvas edge using the gridboxPadding method. All padding values should be positive numbers. If only 'left' is specified then this values sets the padding on all sides of the gridbox. If 'left' and 'bottom' are defined then the right and top padding are set to the same values. If only the top is undefined then top is set equal to the bottom.
NOTE: The Cango property 'cgo.heightPW' holds the height of the canvas as a percentage of the canvas width often useful in setting the gridbox 'top' padding when a fixed height gridbox is required.
If no call is made to gridboxPadding then the default gridbox is the full canvas. Any call to change gridboxPadding resets the world coordinates to their default which is equivalent to calling setWorldCoordsSVG() i.e. the coordinate origin will be the upper left corner of the gridbox and the scaling is 1 to 1 with the canvas pixels.
Parameters:
left: Number - (optional) Width of the padding from left edge of the canvas to the left of the gridbox measured as a percentage of the canvas width. Negative values are replaced with 0.
bottom: Number - (optional) Width of the padding from bottom edge of the canvas to the bottom of the gridbox measured as a percentage of the canvas width. Negative values are replaced with 0.
right: Number - (optional) Width of the padding from right edge of the canvas to the right of the gridbox measured as a percentage of the canvas width. Negative values are replaced with 0.
top: Number - (optional) Width of the padding from top edge of the canvas to the top of the gridbox measured as a percentage of the canvas width. Negative values are replaced with 0.
Syntax:
cgo.fillGridbox(fillColor);
Description:
Fills the current gridbox area with the color fillColor.
Parameters:
fillColor:String or color gradient- The fill color may be solid color defined by a string in one of the CSS color formats or if a gradient color is to be used, a reference to a LinearGradient or RadialGradient object should be passed, see Colors. If 'fillGridbox' is called with no 'fillColor' parameter then the gridbox is filled with the current Cango default fillColor. If not set by the application, the default fillColor is "rgba(128,128,128,1.0)" which is gray.
Syntax:
cgo.setWorldCoordsRHC([gbLowerLeftX, gbLowerLeftY, gbWidth [, gbHeight]]);
Description:
Defines a Right Handed Cartesian coordinate grid for subsequent drawing operations with the cgo graphics context. RHC coordinates have the X axis values increase to the RIGHT and Y axis values increase UP the canvas and angles increase ANTI-CLOCKWISE. The world coordinate system sets translation and scaling factors to map world coordinate values to canvas drawing pixels. This is done by specifying the world coordinate values of the Cango context's gridbox origin and the width and height of the gridbox in world coordinate units. The 'gbLowerLeftX, gbLowerLeftY' will be the world coordinate values of the lower left corner of the gridbox. Specifying the gridbox width in world coordinate units determines the X axis scale factor (cgo.xscl) and specifying the gridbox height in world coordinates Y axis units will determine the Y axis scale factor (cgo.yscl). If no value is passed for the 'gbHeight' then the magnitude of the Y axis scale factor will be set equal to the X axis scale factor resulting in square pixels.
Calling 'setWorldCoordsRHC' with no parameters results in the lower left corner of the gridbox being set to 0,0 in world coordinates and X and Y axes scaled in canvas pixels.
Note: The world coordinate system extends to the full size of the canvas.
Note: If no call has been made to either 'setWorldCoordsRHC' or 'setWorldCoordsSVG', then the world coordinate system will be SVG style equivalent to calling cgo.setWorldCoordsSVG().
Parameters:
gridOrgX: Number - World coordinate X value of the gridbox lower left corner.
gridOrgY: Number - World coordinate Y value of the gridbox lower left corner.
gridboxWidth: Number - The width of the gridbox measured in world X axis units.
gridboxHeight: Number - The height of the gridbox measured in world Y axis units. If 'gbHeight' is omitted then Y axis units are assumed equal to X axis units and so Y scaling is set to equal the X scaling, resulting in square pixels.
Example:
Here is a typical world coordinate setup where gridboxPadding 10% around the gridbox. Then setWorldCoordsRHC fixes the origin (lower left corner) to be 0,0 and the plotting area to be 250 units wide and 3 units high.
function setCoordsDemo(cvsID) { var g = new Cango(cvsID); g.clearCanvas("lightyellow"); g.gridboxPadding(10); g.fillGridbox("lightgreen"); g.setWorldCoordsRHC(0,0, 250,3); g.drawAxes(); }
Syntax:
cgo.setWorldCoordsSVG([gbUpperLeftX, gbUpperLeftY, gbWidth [, gbHeight]]);
Description:
Defines an SVG (Left Handed Cartesian) coordinate grid for subsequent drawing operations with the cgo graphics context. SVG coordinates have the X axis increase to the RIGHT and Y axis increase DOWN the canvas and angles increase CLOCKWISE. This is the sign convention used when drawing with canvas CanvasRenderingContext2D commands. The world coordinate system sets translation and scaling factors to map world coordinate values to canvas drawing pixels. This is done by specifying the world coordinate values of the Cango context's gridbox upper left corner and the width and height of the gridbox in world coordinate units. Specifying the gridbox width in world coordinate units determines the X axis scale factor (cgo.xscl) and specifying the gridbox height in world coordinates Y axis units will determine the Y axis scale factor (cgo.yscl). If no value is passed for the 'gbHeight' then the magnitude of the Y axis scale factor will be set equal to the X axis scale factor resulting in square pixels.
Calling 'setWorldCoordsSVG' with no parameters results in the upper left of the gridbox being set to 0,0 and X and Y axes scaled in canvas pixels.
Note: The world coordinate system extends to the full size of the canvas.
Note: If no call has been made to either 'setWorldCoordsRHC' or 'setWorldCoordsSVG', then the world coordinate system will be SVG style equivalent to calling cgo.setWorldCoordsSVG().
Parameters:
gridOrgX: Number - World coordinate X value of the gridbox upper left corner.
gridOrgY: Number - World coordinate Y value of the gridbox upper left corner.
gridboxWidth: Number - The width of the gridbox measured in world X axis units.
gridboxHeight: Number - The height of the gridbox measured in world Y axis units. If 'gridboxHeight' is omitted then Y axis units are assumed equal to X axis units and so Y scaling is set to equal the X scaling, resulting in square pixels.
Syntax:
cgo.dupCtx(sourceCtx);
Description:
This method copies the graphics context properties from one Cango context 'sourceCtx' into the 'cgo' context. When drawing onto layers, this method provides a quick way to copy the Cango coordinate system for use on the new layer's world coordinates match those on the source context's layer. cgo.dupCtx() copies the properties, scale factors and so on, from the graphics context sourceCtx. Properties copied exclude the 'cvs' and 'cId' as these will have been set when the Cango calling instance 'cgo' was created. Properties are copied by value so that after the call changing the 'sourceCtx' properties will have no effect on 'cgo' properties.
Parameters:
sourceCtx: Cango - The source Cango from which the cgo properties are copied.
Syntax:
var posObj = cgo.toPixelCoords(xWC, yWC);
Description:
This method converts the coordinates (x, y) of a point expressed in world coordinates to an object with x and y properties that hold the raw canvas pixel coordinates of the point. In general canvas pixel coordinates set by 'width' and 'height' attributes may differ from screen pixel coordinates but Cango sets the canvas attributes to match screen pixels when the Cango graphics context is created. So toPixelCoords always returns the canvas pixel coordinates which map 1 to 1 with screen pixels.
Parameters:
xWC, yWC:Numbers - X and Y coordinates of a point measured in world coordinates.
Returns:
Object - {x: , y: } An object with the following properties:
x, y: Numbers - The X and Y coordinates of the point measured in canvas pixel coordinates, equal to screen pixels.
Syntax:
var posObj = cgo.toWorldCoords(xPX, yPX);
Description:
This method converts the coordinates (xPX, yPX) of a point expressed in canvas pixels coordinates to an object with x and y properties that hold the world coordinates of the point. There can be more than one Cango graphics context on a canvas, the world coordinates of the point in the calling graphics context are returned.
Parameters:
xPX, yPX: Numbers - X and Y coordinates of a point measured in pixel coordinates.
Returns:
Object - {x: , y: } An object with two properties x, y which hold the world coordinates the point.
Layer methods
The functionality of a CanvasStack is built into Cango. Transparent canvas overlays can be created to assist in drawing data cursors, and animation.
Layers can be drawn upon and cleared independent of the background canvas or other layers, so animations or drag-n-drop handlers can erase their canvas and re-draw at each frame or move event without affecting the content on other layers.
Note: Detection of a 'grab' event on an object for drag-and-drop works regardless of the layer on which the object is drawn. When a drag-n-drop mouse event occur on a canvas stack, each object enabled for drag-and-drop on each layer is checked to see if the event coordinates are within its outline. The first Obj2D encountered that has drag-n-drop enabled on itself or its parent Group determines the event handler to be called. The layers are searched starting at the top (last canvas added) and going down to the background canvas.
Syntax:
var ovlID = cgo.createLayer();
Description:
Creates a transparent canvas over-lying the background canvas. The overlay canvas will be the same size as the background canvas and will be transparent. A unique ID string is generated and assigned to the new canvas, this ID string is the return value from the method.
Only Cango contexts that are created on the original background canvas can create layers, calling 'createLayer' as a method of a Cango instance on an overlay layer does nothing and returns an empty string.
To draw on the new layer, an instance of Cango should be created on the overlay by calling the Cango constructor passing the layer's ID string. The properties of the background canvas can be readily copied to the new Cango context using the cgo.dupCtx method.
Parameters:
none
Returns:
String - The unique ID string of the newly created canvas element. This ID string allows reference to the canvas overlay to create Cango instances on the layer. The ID string is also required by the Cango 'deleteLayer' method.
Syntax:
cgo.deleteLayer(ovlID);
Description:
Deletes an existing canvas overlay specified by its ID string. The ID of a canvas layer is returned from the 'createLayer' method. The 'deleteLayer' method will remove the layer's canvas from the DOM. The canvas can be anywhere within the stack except the background canvas which cannot be deleted with this method.
Parameters:
ovlID: String - The ID attribute of the canvas element to be deleted.
Syntax:
cgo.deleteAllLayers();
Description:
Deletes all existing canvas layers leaving only the original background canvas. The overlay canvas elements are deleted from the DOM.
Parameters:
none
Basic drawing methods
Cango provides four basic methods for simple, single use, drawing of objects onto the canvas. They are; cgo.drawPath, cgo.drawShape, cgo.drawImg and cgo.drawText. The methods create a corresponding object type and render it to the canvas. The methods take as parameters the object definition and an optional 'options' object which sets various properties that determine the appearance and position of the object on the canvas.
Syntax:
cgo.drawPath(pathData [, options]);
Description:
Creates a Path object defined by the 'pathData' parameter and and sets any of its formatting properties specified by the 'options' parameter and renders the Path onto the canvas. 'pathData' can be a string or an array which holds a series of commands and their associated coordinates in the SVG path data syntax.
Parameters:
pathData: String or Array - A string or array of commands and coordinates in SVG path data format, the full SVG command set is supported (see SVG / Cgo2D syntax for the command descriptions).
options: Object - The various Path object properties can be set by assigning the desired value to the corresponding options property.
Path options properties are:
- x
- y
- scl
- degs
- strokeColor
- lineWidthWC
- lineWidth
- lineCap
- dashed
- dashOffset
- shadowOffsetX
- shadowOffsetY
- shadowBlur
- shadowColor
(see Path.setProperty method for more details).
Syntax:
cgo.drawShape(pathData [, options]);
Description:
Creates a Shape defined by the 'pathData' parameter and and sets any of its formatting properties specified by the 'options' parameter and renders it onto the canvas. 'pathData' can be a string or an array holding a series of commands and their associated coordinates in the SVG path data syntax. The outline path is closed and the Shape formed is filled with color. The 'options' object (if present) can specify where the Shape is positioned and how it is formatted when rendered.
Parameters:
pathData: String or Array - A string or array of commands and coordinates in SVG path data format, the full SVG command set is supported (see SVG / Cgo2D syntax for the command descriptions).
options: Object - The various Shape object properties can be set by assigning the desired value to the corresponding options property.
Shape options properties are:
- x
- y
- scl
- degs
- fillColor
- strokeColor
- lineWidthWC
- lineWidth
- dashed
- dashOffset
- border
- shadowOffsetX
- shadowOffsetY
- shadowBlur
- shadowColor
(see Shape.setProperty method for more details).
Syntax:
cgo.drawImg(imgSpec [, options]);
Description:
Renders an Image specified by 'imgSpec' onto the canvas. The imgSpec can be a URL string or a pre-loaded Image object. The properties of the 'options' object (if present) can specify where the image is positioned and how it is formatted when rendered.
Parameters:
imgSpec: Image or String - The pre-loaded Image object or the URL of the image to be loaded and then rendered to the canvas.
options: Object - The various Img object properties can be set by assigning the desired value to the corresponding options property.
Img options properties are:
- x
- y
- scl
- degs
- strokeColor
- lineWidthWC
- lineWidth
- dashed
- dashOffset
- border
- imgWidth
- imgHeight
- lorg
- shadowOffsetX
- shadowOffsetY
- shadowBlur
- shadowColor
(see Img.setProperty method for more details).
Syntax:
cgo.drawText(str [, options]);
Description:
Renders a Text object onto the canvas. The text is specified by the string 'str'. The 'options' object (if present) can specify where the Text is positioned and how it is formatted when rendered.
Parameters:
str: String - The text string to be written.
options: Object - The various Text object properties can be set by assigning the desired value to the corresponding options property.
Text options properties are:
- x
- y
- scl
- degs
- strokeColor
- fillColor
- lineWidthWC
- lineWidth
- dashed
- dashOffset
- border
- fontFamily
- fontSize
- fontWeight
- lorg
- shadowOffsetX
- shadowOffsetY
- shadowBlur
- shadowColor
(see Text.setProperty method for more details).
Syntax:
cgo.clearCanvas([fillColor]);
Description:
Clears the canvas, deleting all drawing, images and text. The canvas may be optionally filled with the color fillColor. If 'clearCanvas' is called 'fillColor' undefined, or 'fillColor' is null, then the canvas is cleared back to its default color which is inherited from the canvas element style. All graphics drawing contexts are left intact. Listening for mousedown events on any draggable objects is canceled.
Parameters:
fillColor: String or color gradient - An optional fill color defined by a string in one of the CSS color formats or if a gradient color is to be used a reference to a LinearGradient or RadialGradient object should be passed, see Colors section for detailed syntax.
Syntax:
cgo.setPropertyDefault(propertyName, val);
Description:
Sets the default values for various properties that will be used if the user code doesn't explicitly set them. The property whose default is to be set is specified by the string 'propertyName' this string is not case sensitive and may take the values set out in column 1 of the table below.
The new default value is passed as 'value'. The value is restricted to be within the range of values appropriate to the property. If the property is not one of the string values listed or the value is an incorrect type or is outside the allowed range of values then the call is ignored and the current default value remains unaltered.
If no call is made to 'setPropertyDefault' for a particular property, then the property value remains at its system default value listed in column 4.
Name | Type | Range | Default |
---|---|---|---|
fillColor | CSS Color | - | "rgba(0, 0, 0, 1.0)" |
strokeColor | CSS Color | - | "rgba(128, 128, 128, 1.0)" |
lineWidth | Number (pixels) | - | 1 |
lineCap | String | "butt", "round", "square" | "butt" |
fontFamily | String | CSS font family definitions | "Consolas, Monaco, 'Andale Mono', monospace" |
fontSize | Number (pixels) | >0 | 12 |
fontWeight | String or Number | "bold", "normal", 100, 200, ..900 | 400 |
stepTime | Number (msec) | 16 .. 500 | 50 |
NB: fontSize pixel value is converted to the equivalent world coordinate value so that fontSize can be scaled with transform methods.
Parameters:
propertyName: String- The name of the property whose default value is to be set. It must be one of the strings listed in column 1 of the table above. The string value is not case sensitive.
value- The new default value for the property. Its type should match the type listed in column 2 of the table above and the range of values must fall within the range listed in column 3.
Objects
For more control of objects as is required for animation or drag-and-drop, objects amy be created independent of a Cango instance and have transforms applied to them and their properties set or modified prior to rendering. The prototype object of all objects types is an Obj2D this is a private object, the five public objects are sub-types of Obj2D. These are created using the constructors: Path, Shape, Img,Text and ClipMask. Once instantiated these Obj2D may be rendered and transformed and re-rendered without having to be re-constructed each time which is desirable in animations. An object, once created can be rendered by different Cango instances on any layer.
All the Obj2D constructors take a definition parameter which may be an outline path definition for Path, Shape and ClipMask, or a URL or HTML Image for Img objects or a string for Text objects. The constructors can take a second parameter that sets the initial values of their properties.
All Obj2D have methods which apply transforms to the object definition. These are; obj.translate, obj.rotate, obj.scale and obj.skew. These are 'hard' transforms which permanently alter the definition of the object. They also all have a 'transform' property whose methods make apply 'soft' ie. temporary transformations to the object as it is rendered. This 'soft' transform matrix is reset after the Obj2D is rendered.
Preserving Aspect Ratio
The Cango graphics context allows independent X and Y axis world coordinate scaling. Different X and Y scaling never distorts text or images and applications usually want Shapes to have their aspect ration preserved. But plotting a graph uses a Path object to hold the data and the X and Y coordinates are usually required vastly different scaling. To give complete control of the aspect ratio Path, Shape and ClipMask objects have an 'iso' property. Setting the obj.iso property to the string 'iso', or the boolean true will ensure that the aspect ratio is preserved independent of differing X and Y world coordinates (X axis scaling is used for both X and Y coordinates). Setting obj.iso false will allow different X and Y world coordinate scaling to be applied when rendered. By default and Shape and ClipMask objects have 'iso' set true and Path objects have iso set false.
Object Drawing origin
The outline of Path, Shape and ClipMask objects are expressed in world coordinates referenced to the drawing origin 0,0. This is their drawing origin. If a obj.translate(x, y) transform is applied the objects drawing origin remains at 0,0 but the object moves by an amount x, y when drawn i.e. effectively x has been added to x coordinates of the path and y to all the y coordinates. If a soft translate is applied obj.transform. translate(x,y) then the drawing origin (0,0) is effectively moved to (x,y) and the object is drawn displaced from the world coordinate origin by x,y. Similarly, Text and Img objects are drawn relative to the drawing origin 0,0. The actual point within the image or text bounding box that is positioned at (0,0) is determined by the objects lorg (locate origin) property. The 'lorg' property can take values from 1..9, where 1=top left, 2=top center, and so on to 9=bottom right. When a obj.translate(x,y) is applied the Image or Text is moved relative to its drawing origin, i.e. the lorg value has been over-ridden, shifted by x,y. If an obj.transform.translate(x,y) is applied then it is the objects 'drawing origin' that is positioned at x,y.
Drop shadows
Any Cango object may be drawn with a drop shadow. All objects support drop shadows defined by properties shadowOffsetX, shadowOffsetY, shadowBlur and shadowColor. By default the shadow dimensions are set to 0 so no shadow is drawn. All dimensions are measured in world coordinates. The drop shadow properties can be set by assigning key-value pairs to the constructor's 'options' parameter or by using the obj.setProperty method. Drop shadow dimension only scale with world coordinate changes (eg. zoom and pan) they are not altered by obj.scale or cgo.render dynamic scaling.
Object Borders
Any Shape, Text or Img object will be drawn with a border if the obj.border property is set true. The color of the border are set by strokeColor property. The width of the border is determined by the objects line width value when rendered. Setting line width is discussed below.
Line width
A Path object's outline and the border width of other object types is drawn with line width set by either the obj.lineWidthWC or the obj.lineWidth property. lineWidthWC sets line width in world coordinate units, lineWidth set line width in pixel units. Two methods of setting the line width are provided to clarify the behaviour of line width when an object is scaled.
There are three methods of scaling the size of an object: 1. The obj.scale method, which actually changes outline coordinates or Image dimensions used to draw the object. 2. The obj.transform.scale method, which will dynamically scale the object as it is drawn. This dynamic scaling will not change the object definition. 3. The Zoom-and-Pan utility can change the rendered size of every object drawn on a canvas by changing the world coordinates of the Cango instance and then re-drawing all the objects.
If a value is assigned to the lineWidthWC property, the line width or border width will scale just like any other dimension of the object with all three scaling methods. If the lineWidthWC property is null or undefined and a value has been assigned to the lineWidth property then Path outline or Shape, Image and Text borders will have this fixed value in pixels as its width regardless of any type of scaling applied to the path or object.
If both properties have been assigned a value then the lineWidthWC takes precedence. If neither has been specified, then the current default value of the Cango.lineWidth (pixel value) belonging to the Cango context used to render the object, is used. This default value may be changed with the Cango.setPropertyDefault method.
Here are more details about each Obj2D type.
Path constructor
Syntax:
var obj = new Path(data[, options]);
Description:
This function creates a Path object suitable to be passed to the 'render' method of a Cango instance. The Path outline is defined by the 'data' parameter which may be an array or a string containing a series of SVG syntax command letters and their associated coordinates.
NOTE: If the string of SVG data is copied from an SVG editor such as Inkscape the Path will appear inverted if the Cango context rendering the Path has a RHC world coordinates (see setWorldCoordsRHC).
The Path outline will be rendered with line width set by 'lineWidth' in pixels or 'lineWidthWC' in world coordinates. The line color is set by the 'strokeColor' property.
Parameters:
data: String or Array - holding a series of commands and their associated coordinates. The full SVG set of commands is supported (see SVG / Cgo2D syntax).
options: Object - An optional JavaScript object containing key-value pairs to set the properties of the Path object. The keys and value ranges are described in the setProperty method.
Example
The following line of code will create a 'Path' object. When rendered 'obj' will be drawn as a square with width and height 4 units in the world coordinate units. The outline will be 2 pixels wide and colored 'red'.
var obj = new Path(['M',2,-2,'l',0,4,-4,0,0,-4,'z'], {lineWidth: 2, strokeColor: 'red'});
Path properties
Property Name | Type | Range / Description |
---|---|---|
strokeColor | CSS Color | see Colors |
lineWidthWC | Number (World Coordinates) | > 0 |
lineWidth | Number (pixels) | > 0 |
lineCap | String | 'butt', 'round', 'square' |
iso | Boolean or String | true, 'iso' or 'isotropic' will preserve the aspect ratio of a Path in non-isotropic coordinates. false will allow independent X and Y scaling. Path default iso value is false. |
dashed | Array | [mark, space ...] the repeating mark-space pixel lengths |
dashOffset | Number (pixels) | length of initial space before the first dash |
shadowOffsetX | Number (World Coordinates) | |
shadowOffsetY | Number (World Coordinates) | |
shadowBlur | Number (World Coordinates) | |
shadowColor | CSS Color | see Colors |
Path methods
Syntax:
obj1.appendPath(obj2 [, delMove]);
Description:
appendPath extends the array of draw commands defining the outline of obj1 by appending the draw commands of obj2. If the optional 'delMove' parameter is 'true', then the initial 'moveTo' command is deleted from the array of commands to be appended.
Parameters:
obj2:Path or Shape object - The Path or Shape whose draw commands are to be appended to the draw commands of obj1.
delMove: Boolean - If 'true' the initial 'moveTo' command of obj2 path outline is omitted. This results in the outline path of obj1 being joined to the outline of obj2. If 'delMove' evaluates to false, then the 'moveTo' command and its coordinates just become the next command in the outline of obj1 which means there will be a break in the outline.
Syntax:
obj.revWinding();
Description:
revWinding re-arranges the order of the draw commands and their coordinates that define the Path's outline so that they are drawn by traversing the outline path in the reverse direction. This method is provided to enable Shapes with holes to be color filled correctly ie. if the hole is traversed in the opposite direction to the container Shape then the hole will not be color filled. The canvas specifications describe the behaviour as follows: "if two overlapping but otherwise independent sub-paths have opposite windings, they cancel out and result in no fill. If they have the same winding, that area just gets painted once". A good explanation of the effects of winding rules is given at BIT-101.com. See Shape.revWinding for example code.
Parameters:
none.
Syntax:
var newObj = obj.dup();
Description:
dup creates a new Path object and copies all the properties and methods from the original into the new object. The properties are copies of the values not references to the original. This method is useful in creating complex objects made from similar components.
Parameters:
none.
Syntax:
obj.setProperty(propertyName, val);
Description:
Sets the values for various Path object properties.
Each property has a Cango default value, so if the property has not been set prior to rendering, then the Cango default value will be used, see setPropertyDefault.
Parameters:
propertyName: String - The Path property whose value is to be set. The string is not case sensitive and may take the values set out in column 1 of the Path properties table above. If the property is not one of these strings the call is ignored.
val: various types - The new value for the property. The value must be of an appropriate type and fall within the useful range of values for the property. The types are listed in column 2 of the Path property table and range of values is listed in column 3, otherwise the call is ignored and the current value remains unaltered.
Syntax:
obj.translate(x, y);
Description:
Adds the values x and y to all draw commands coordinates of the Path object. Effectively making a permanent shift of the object by x and y from the drawing origin. The change is permanent, the center of rotation and scaling will be about this new drawing origin.
Parameters:
x, y: Numbers - X and Y offset of the Path object from the current drawing origin.
Syntax:
obj.rotate(degs);
Description:
Rotates the Path by 'degs' degrees centered on the current drawing origin. The change to the orientation of the Path is permanent, when the Path is rendered the new angle will be the reference for future rotation transforms.
Parameters:
degs: Number - The angle of rotation measured in degrees counter-clockwise for RHC coordinate grids and clockwise for SVG coordinate grids.
Syntax:
obj.scale(xScale[, yScale]);
Description:
Changes the dimensions of the Path object by multiplying X dimensions by 'xScale' and Y dimensions by 'yScale' (if specified). If 'yScale' is undefined then 'xScale' value is used to scale the Y dimensions. This scaling permanently changes the size of the Path object as it will be rendered to the canvas. The scaling is centered on the drawing origin.
If obj.lineWidthWC is defined then the line width of the Path or the width of any border will be scaled by 'xScale'. If the lineWidthWC is undefined then the line width is set by the lineWidth property (in pixels) will remain unaffected by this scale method.
Parameters:
xScale: Number - A number by which the object's X dimensions are scaled, negative numbers will flip the object horizontally if the num.
yScale: Number - (optional) A number by which the object's Y dimensions are scaled, negative numbers will flip the object vertically.
Syntax:
obj.skew(degH, degV);
Description:
Applies a transform to skew the path outline X coordinates by an angle of 'degH', and skewing the Y coordinates by an angle of 'degV'.
Parameters:
degH: Number - Skew angle measured in degrees counter-clockwise from the X axis.
degH, degV: Numbers - Skew angle measured in degrees counter-clockwise from the Y axis.
Path objects like all Obj2D and Group have a 'transform' property which consists of a matrix holding the geometric transforms to be applied to the object when it is rendered to the canvas. The transform matrix is built up by successive calls to the obj.transform methods. These transforms do not affect the object definition, the transform matrix is reset to the identity matrix after the Path object is rendered. The supported methods are:
obj.transform.translate(xOfs, yOfs); obj.transform.scale(xScl, yScl); obj.transform.rotate(degs); obj.transform.skew(degH, degV); obj.transform.revolve(deg);
see obj.transform methods for details.
Syntax:
obj.enableDrag(grabCallback, dragCallback, dropCallback);
Description:
This method creates an object type Drag2D which is assigned to the obj.dragNdrop property. Drag2D objects encapsulate the event handlers that run when mousedown, mousemove and mouseup events occur within the outline of the Path as drawn on the canvas. The event handlers call the user defined callback functions passed to 'enableDrag'. 'grabCallback' is called on a mousedown events, 'dragCallback' and 'dropCallback' functions are called on subsequent mousemove and mouseup or mouseout events. The current cursor location is passed to the callback functions as an object with properties 'x' and 'y' holding the cursor coordinates measured in world coordinates. The obj will have drag and drop capability enabled whenever it is rendered to the canvas.
To simplify writing grab, drag and drop functions, the callbacks are executed in the scope of a Drag2D object which has properties to supply these values. All the handy properties in scope of the handlers are listed in the Drag2D section below.
When drag-n-drop is enabled on 'obj' the canvas mousedown event listener is armed to check for mousedown events within its outline. As long as the obj.dragNdrop property is not 'null', the mousedown listener is activated every time 'obj' is rendered to the canvas. The drag-n-drop is de-activated if the canvas is cleared by a call to cgo.clearCanvas, or by calling the obj.disableDrag method.
Parameters:
grabCallback: Function or null - This function to be called when a 'mousedown' event occurs within the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dragCallback: Function or null - This function to be called when a 'mousemove' event occurs after the mouse down event has occurred with the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dropCallback: Function or null - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
Syntax:
obj.disableDrag();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of Obj2D to be checked for a hit on mousedown events.
Parameters:
none
Shape constructor
Syntax:
var obj = new Shape(data[, options]);
Description:
This function returns a Shape object suitable to be passed to the 'render' method of a Cango instance. The Shape outline is defined by the 'data' parameter which may be an array or a string containing a series of SVG syntax command letters and their associated coordinates. If the Shape outline is not closed by a final 'Z' command it is automatically added to close the Shape before it is rendered and color filled. The color is set by the value of its 'fillColor' property.
NOTE: If the string of SVG data is copied from an SVG editor such as Inkscape the Shape will appear inverted if the Cango context rendering the Shape has RHC world coordinates (see setWorldCoordsRHC).
Parameters:
data: String or Array - A string or an array holding a series of commands and their associated coordinates. The full SVG set of commands is supported (see SVG / Cgo2D syntax).
options: Object - This object may contain key-value pairs to pre-set the properties of the object returned. The keys and value restrictions are identical to those described in the setProperty method.
Example
The following line of code will create a Shape object and then scale its size. When rendered 'obj' will be drawn as a square with width and height 4 units in the world coordinates of the Cango context used to render it.
var obj = new Shape(['M',2,-2,'l',0,4,-4,0,0,-4,'z'], {fillColor: 'pink'});
Shape properties
Property Name | Type | Range / Description |
---|---|---|
fillColor | CSS Color or gradient | see Colors |
strokeColor | CSS Color | see Colors |
lineWidthWC | Number (World Coordinates) | > 0, width of a border |
lineWidth | Number (pixels) | > 0, width of a border |
iso | Boolean or String | true, 'iso' or 'isotropic' will preserve the aspect ratio of a Shape in non-isotropic coordinates. false will allow independent X and Y scaling. Shape default iso value is true. |
dashed | Array | [mark, space ...] the repeating mark-space pixel lengths of dashed border |
dashOffset | Number (pixels) | length of initial space before the first dash of dashed border |
border | Boolean | true or false |
shadowOffsetX | Number (World Coordinates) | |
shadowOffsetY | Number (World Coordinates) | |
shadowBlur | Number (World Coordinates) | |
shadowColor | CSS Color | see Colors |
Shape methods
Syntax:
obj1.appendPath(obj2 [, delMove]);
Description:
appendPath extends the array of draw commands defining the outline of 'obj1' by appending the draw commands of 'obj2'. If the optional 'delMove' parameter is 'true', then the initial 'moveTo' command is deleted from the array of commands to be appended.
Parameters:
obj2: Path or Shape object - The Path or Shape object whose drawCmd array elements are to be appended to the drawCmd array of obj1.
delMove: Boolean - If 'true' the initial 'moveTo' command of obj2 path outline is omitted. This results in the outline path of obj1 being joined to the outline of obj2. If 'delMove' evaluates to false, then the 'moveTo' command and its coordinates just become the next command in the outline of obj1 which means the appended outline will form a separate closed Shape.
Syntax:
obj.revWinding();
Description:
revWinding re-arranges the order of the draw commands and their coordinates that define the outline path of this Shape so that they are drawn by traversing the path in the reverse direction. This command is provided to enable Shapes with holes to be color filled correctly ie. without the hole being filled too. The canvas specifications describe the behaviour as follows: "if two overlapping but otherwise independent sub-paths have opposite windings, they cancel out and result in no fill. If they have the same winding, that area just gets painted once". A good explanation of the effects of winding rules is given at BIT-101.com.
Parameters:
none.
Example
As an example, the following code attempts (and fails) to draw a triangle with a circular hole at the center:
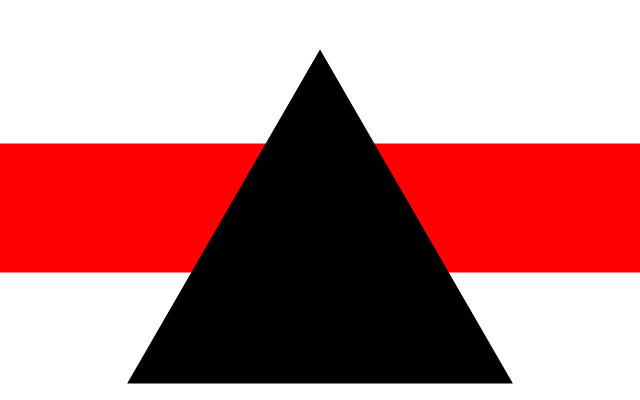
var g = new Cango(cvsID); ... var rect = ["M", 0, 100, 500, 100, 500, 200, 0, 200, "z"], tri = new Shape(shapeDefs.triangle(300), {fillColor: 'black'}), hole = new Shape(shapeDefs.circle(140)); tri.appendPath(hole); tri.translate(250, 100); grp = new Group(rect, tri); g.render(grp);
If the winding direction of the 'hole' Path is reversed before appending to the triangle, the hole is not filled:
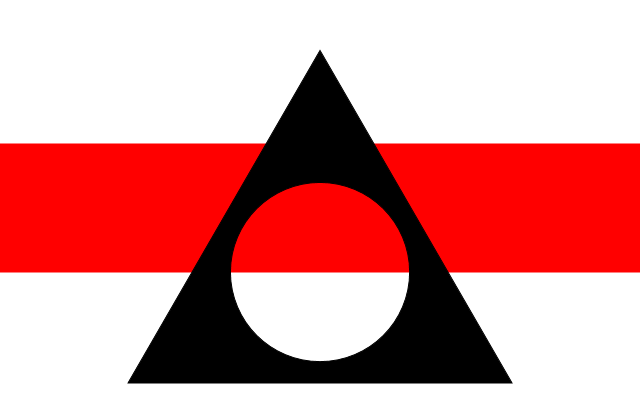
var g = new Cango(cvsID); ... var rect = ["M", 0, 100, 500, 100, 500, 200, 0, 200, "z"], tri = new Shape(shapeDefs.triangle(300), {fillColor: 'black'}), hole = new Shape(shapeDefs.circle(140)); hole.revWinding(); // reverse winding for this object tri.appendPath(hole); tri.translate(250, 100); grp = new Group(rect, tri); g.render(grp);
Syntax:
var newObj = obj.dup();
Description:
dup creates a new Shape object and copies all the properties and methods from the original into the new object. The properties are copies of the values not references to the original. This method is useful in creating complex objects made from similarly shaped components.
Parameters:
none.
Syntax:
obj.setProperty(propertyName, val);
Description:
Sets the values for various Shape object properties.
Each property has a Cango default value, so if the property has not been set prior to rendering, then the Cango default value will be used, see setPropertyDefault.
Parameters:
propertyName: String - The Shape property whose value is to be set. The string is not case sensitive and may take the values set out in column 1 of the Shape properties table above. If the property is not one of these strings the call is ignored and the current value remains unaltered.
val: various types - The new value for the property. The value must be of an appropriate type and fall within the useful range of values for the property. The types are listed in column 2 of the Shape property table and range of values is listed in column 3, otherwise the call is ignored and the current value remains unaltered.
Syntax:
obj.translate(x, y);
Description:
Adds the values x and y to all draw commands coordinates of the Shape outline. Effectively making a permanent shift of the Shape by x and y relative to its drawing origin. The change to the drawing origin is permanent, the center of rotation and scaling will be about this new drawing origin.
Parameters:
x, y: Numbers - X and Y offset of the object from the current drawing origin.
Syntax:
obj.rotate(degs);
Description:
Rotates the Shape object by 'degs' degrees centered on the current drawing origin. The change to the orientation of the object is permanent, when the Shape is rendered the new angle will be the reference for future rotation transforms.
Parameters:
degs: Number - The angle of rotation measured in degrees counter-clockwise for RHC coordinate grids and clockwise for SVG coordinate grids.
Syntax:
obj.scale(xScale[, yScale]);
Description:
Changes the dimensions of the Shape by multiplying the outline path X coordinates by 'xScale' and Y coordinates by 'yScale' (if specified). If 'yScale' is omitted or invalid then 'xScale' value is used to scale the Y coordinates. This scaling permanently changes the size of the Shape object as it will be rendered to the canvas. The scaling is centered on the drawing origin.
If obj.lineWidthWC is defined and border set 'true' then the width of the border will be scaled by 'xScale'. If the lineWidthWC is undefined then the width used for the border will remain unaffected by this scale method.
Parameters:
xScale: Number - A number by which the object's X dimensions are scaled, negative numbers will flip the object horizontally if the num.
yScale: Number - (optional) A number by which the object's Y dimensions are scaled, negative numbers will flip the object vertically.
Syntax:
obj.skew(degH, degV);
Description:
Applies a transform to skew the shape outline path X coordinates by an angle of 'degH', and skewing the Y coordinates by an angle of 'degV'.
Parameters:
degH: Number - Skew angle measured in degrees counter-clockwise from the X axis.
degH, degV: Numbers - Skew angle measured in degrees counter-clockwise from the Y axis.
Shape objects like all Obj2D and Group ave a 'transform' property which consists of a matrix holding the geometric transforms to be applied to the Shape when it is rendered to the canvas. The transform matrix is built up by calling the obj.transform methods. These transforms do not affect the Shape definition, the transform matrix is reset to the identity matrix after the Shape is rendered. The supported methods are:
obj.transform.translate(xOfs, yOfs); obj.transform.scale(xScl, yScl); obj.transform.rotate(degs); obj.transform.skew(degH, degV); obj.transform.revolve(deg);
see obj.transform methods for details.
Syntax:
obj.enableDrag(grabCallback, dragCallback, dropCallback);
Description:
This method creates an object type Drag2D which is assigned to the obj.dragNdrop property. Drag2D objects encapsulate the event handlers that run when mousedown, mousemove and mouseup events occur within the outline of the Shape as drawn on the canvas. The event handlers call the user defined callback functions passed to 'enableDrag'. 'grabCallback' is called on a mousedown events, 'dragCallback' and 'dropCallback' functions are called on subsequent mousemove and mouseup or mouseout events. The current cursor location is passed to the callback functions as an object with properties 'x' and 'y' holding the cursor coordinates measured in world coordinates. The obj will have drag and drop capability enabled whenever it is rendered to the canvas.
To simplify writing grab, drag and drop functions, the callbacks are executed in the scope of a Drag2D object which has properties to supply these values. All the handy properties in scope of the handlers are listed in the Drag2D section below.
When drag-n-drop is enabled on 'obj' the canvas mousedown event listener is armed to check for mousedown events within its outline. As long as the obj.dragNdrop property is not 'null', the mousedown listener is activated every time 'obj' is rendered to the canvas. The drag-n-drop is de-activated if the canvas is cleared by a call to cgo.clearCanvas, or by calling the obj.disableDrag method.
Parameters:
grabCallback: Function or null - This function to be called when a 'mousedown' event occurs within the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dragCallback: Function or null - This function to be called when a 'mousemove' event occurs after the mouse down event has occurred with the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dropCallback: Function or null - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
Syntax:
obj.disableDrag();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of Obj2D to be checked for a hit on mousedown events.
Parameters:
none
Img constructor
Syntax:
var obj = new Img(data[, options]);
Description:
This function returns a Img object suitable to be passed to the 'render' method of a Cango instance.
Img objects take an image file URL or an HTML Image object as a parameter. If the URL is passed then the image is loaded into an HTML Image object and properties describing how it is to be drawn are stored ready to be rendered onto the canvas. Image width and height may be set independently in world coordinate units using the obj.imgWidth and obj.imgHeight properties or may be drawn with the image aspect ratio preserved by setting only one of imgWidth or imgHeight.
Parameters:
data: HTML Image object or String - The definition of the image to be drawn. This can be a reference to a pre-loaded Image object or a string specifying the URL from which the image file can be loaded.
options: Object - This object may contain key-value pairs to pre-set the properties of the Img object returned. The keys and value restrictions are identical to those described in the setProperty method.
Example
The following line of code will initiate the loading of the file "JaneAvril.jpg" from the "Images" subdirectory. When the load completes the image will be available for rendering to the canvas. When rendered 'jane' object will be drawn 100 world coordinate units wide with aspect ratio preserved. It will be given a red border 4 pixels wide. When rendered the image drawing origin will be the center-top of the image (lorg=2). This drawing origin point will be rendered at the world coordinate origin unless jane.transform.translate(x,y) method is called prior to rendering in which case the image center-top will be placed at (x,y).
var jane = new Img("Images/JaneAvril.jpg", { imgHeight: 100, lorg: 2, border: true, lineWidth: 4, strokeColor: "ff8020" });
Img properties
Property Name | Type | Range / Description |
---|---|---|
strokeColor | CSS Color | Specifies border color, see Colors |
lineWidthWC | Number (World Coordinates) | > 0, width of a border |
lineWidth | Number (pixels) | > 0, width of a border |
dashed | Array | [mark, space ...] the repeating mark-space pixel lengths of a dashed border |
dashOffset | Number (pixels) | length of initial space before the first dash of a dashed border |
border | Boolean | true or false |
imgWidth | Number (World Coordinates) | >0 |
imgHeight | Number (World Coordinates) | >0 |
lorg | Number | 1..9 (see lorg) |
shadowOffsetX | Number (World Coordinates) | |
shadowOffsetY | Number (World Coordinates) | |
shadowBlur | Number (World Coordinates) | |
shadowColor | CSS Color | see Colors |
Img methods
Syntax:
obj.setProperty(propertyName, val);
Description:
Sets the values for various Img object properties.
Each property has a Cango default value, so if the property has not been set prior to rendering, then the Cango default value will be used, see setPropertyDefault.
Parameters:
propertyName: String - The Img property whose value is to be set. The string is not case sensitive and may take the values set out in column 1 of the Img properties table above. If the property is not one of these strings the call is ignored and the current value remains unaltered.
val: various types - The new value for the property. The value must be of an appropriate type and fall within the useful range of values for the property. The types are listed in column 2 of the Img property table and range of values is listed in column 3, otherwise the call is ignored and the current value remains unaltered.
Syntax:
var newObj = obj.dup();
Description:
dup creates a new Img object and copies all the properties and methods into the new object. The new Img will always render the same Image object, but the other properties are copied by value not reference.
Parameters:
none.
Syntax:
obj.translate(x, y);
Description:
Applies a hard 'translate' matrix transform to the Img object. Effectively making a permanent shift of the Img object relative to its drawing origin. The center of rotation and scaling will be about this new drawing origin. The drawing origin of Img object is initially set by the 'lorg' parameter to a restricted number of positions on the image, translate allows the Img to be arbitrarily offset from the drawing origin.
Parameters:
x, y: Numbers - X and Y offset of the Img relative to its drawing origin.
Syntax:
obj.rotate(degs);
Description:
Rotates the Img by 'degs' degrees centered on the current drawing origin. The change to the orientation is permanent, when the Img object is rendered the new angle will be the reference for future rotation transforms.
Parameters:
degs: Number - The angle of rotation measured in degrees counter-clockwise for RHC coordinate grids and clockwise for SVG coordinate grids.
Syntax:
obj.scale(xScale[, yScale]);
Description:
Changes the dimensions of the Img by multiplying width by 'xScale' and height by 'yScale' (if specified). If 'yScale' is undefined then 'xScale' value is used to scale the both width and height. This scaling permanently changes the size of the object as it will be rendered to the canvas. The scaling is centered on the drawing origin so the object doesn't shift position, just expands or contracts about the drawing origin.
If obj.lineWidthWC is defined then the width of any border will be scaled by 'xScale'. If the lineWidthWC is undefined then the line width used for borders will remain unaffected by this scale method.
Parameters:
xScale: Number - A number by which the object's X dimensions are scaled, negative numbers will flip the image horizontally if the num.
yScale: Number - (optional) A number by which the object's Y dimensions are scaled, negative numbers will flip the image vertically.
Syntax:
obj.skew(degH, degV);
Description:
Applies a 'hard' transform matrix to skew the image by an angle of 'degH' from the X axis, and by an angle of 'degV' from the Y axis.
Parameters:
degH: Number - Skew angle measured in degrees counter-clockwise from the X axis.
degH, degV: Numbers - Skew angle measured in degrees counter-clockwise from the Y axis.
Img objects like all Obj2D and Group ave a 'transform' property which consists of a matrix holding the geometric transforms to be applied to the object when it is rendered to the canvas. The transform matrix is built up by calling the obj.transform methods. These transforms do not affect the object definition, the transform matrix is reset to the identity matrix after the Img object is rendered. The supported methods are:
obj.transform.translate(xOfs, yOfs); obj.transform.scale(xScl, yScl); obj.transform.rotate(degs); obj.transform.skew(degH, degV); obj.transform.revolve(deg);
see obj.transform methods for details.
Syntax:
obj.enableDrag(grabCallback, dragCallback, dropCallback);
Description:
This method creates an object type Drag2D which is assigned to the obj.dragNdrop property. Drag2D objects encapsulate the event handlers that run when mousedown, mousemove and mouseup events occur within the outline of the Img as drawn on the canvas. The event handlers call the user defined callback functions passed to 'enableDrag'. 'grabCallback' is called on a mousedown events, 'dragCallback' and 'dropCallback' functions are called on subsequent mousemove and mouseup or mouseout events. The current cursor location is passed to the callback functions as an object with properties 'x' and 'y' holding the cursor coordinates measured in world coordinates. The obj will have drag and drop capability enabled whenever it is rendered to the canvas.
To simplify writing grab, drag and drop functions, the callbacks are executed in the scope of a Drag2D object which has properties to supply these values. All the handy properties in scope of the handlers are listed in the Drag2D section below.
When drag-n-drop is enabled on 'obj' the canvas mousedown event listener is armed to check for mousedown events within its outline. As long as the obj.dragNdrop property is not 'null', the mousedown listener is activated every time 'obj' is rendered to the canvas. The drag-n-drop is de-activated if the canvas is cleared by a call to cgo.clearCanvas, or by calling the obj.disableDrag method.
Parameters:
grabCallback:Function or null - This function to be called when a 'mousedown' event occurs within the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dragCallback:Function or null - This function to be called when a 'mousemove' event occurs after the mouse down event has occurred with the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dropCallback:Function or null - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
Syntax:
obj.disableDrag();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of Obj2D to be checked for a hit on mousedown events.
Parameters:
none
Text constructor
Syntax:
var obj = new Text(data[, options]);
Description:
This function returns a Text object suitable to be passed to the 'render' method of a Cango instance.
Text objects take a string parameter which can be drawn in any of the CSS fonts available to the system, with user defined font size and weight etc.
Parameters:
data: String - The 'data' string will be the actual text to be rendered to the canvas.
options: Object - This object may contain key-value pairs to pre-set the properties of the Text object returned. The keys and value restrictions are identical to those described in the setProperty method.
Example
var obj = new Text("Hullo World", { fillColor: "blue", fontSize: 28, lorg:5 });
This code snippet will create a Text object when rendered, the text "Hullo World" will be drawn onto the canvas. The fontSize will be 28 pixels, fontWeight and fontFamily will be the default values of Cango context used to render the Text as these properties have not been explicitly set. The text will be colored blue. The lorg 5 value will result in drawing origin being set at the center of the text bounding box. When rendered the drawing origin will be placed at the world coordinate origin (0,0) unless a translate transform is applied to the Text prior to rendering.
Text properties
Property Name | Type | Range / Description |
---|---|---|
strokeColor | CSS Color | Specifies border color, see Colors |
fillColor | CSS Color or gradient | Specifies text color, see Colors |
lineWidthWC | Number (World Coordinates) | > 0, width of border |
lineWidth | Number (pixels) | > 0, width of border |
dashed | Array | [mark, space ...] the repeating mark-space pixel lengths of a dashed border |
dashOffset | Number (pixels) | length of initial space before the first dash of a dashed border |
border | Boolean | true or false |
fontFamily | String | CSS fontFamily definitions |
fontSize | Number (pixels) | - |
fontWeight | String or Number | "bold", "normal", 100, 200, ..900 |
lorg | Number | 1 .. 9 (see lorg) |
shadowOffsetX | Number (World Coordinates) | |
shadowOffsetY | Number (World Coordinates) | |
shadowBlur | Number (World Coordinates) | |
shadowColor | CSS Color | see Colors |
Text methods
Syntax:
obj.setProperty(propertyName, val);
Description:
Sets the values for various Text object properties.
Each property has a Cango default value, so if the property has not been set prior to rendering, then the Cango default value will be used, see setPropertyDefault.
Parameters:
propertyName: String - The Text property whose value is to be set. The string is not case sensitive and may take the values set out in column 1 of the Text properties table above. If the property is not one of these strings the call is ignored and the current value remains unaltered.
val: various types - The new value for the property. The value must be of an appropriate type and fall within the useful range of values for the property. The types are listed in column 2 of the Text property table and range of values is listed in column 3, otherwise the call is ignored and the current value remains unaltered.
Syntax:
var newObj = obj.dup();
Description:
dup creates a new Text object and copies all the properties and methods from 'obj' into the new object. The properties are copied by value not reference.
Parameters:
none.
Syntax:
obj.translate(x, y);
Description:
Applies a hard 'translate' matrix transform to the Text object. Effectively making a permanent shift of the Text relative to its drawing origin. The center of rotation and scaling will be about this new drawing origin. The drawing origin of Text object is initially set by the 'lorg' parameter to a restricted number of positions on the text bounding box, translate allows the Text to be arbitrarily offset from the drawing origin.
Parameters:
x, y: Numbers - X and Y offset of the Text relative to its current drawing origin.
Syntax:
obj.rotate(degs);
Description:
Rotates the Text object by 'degs' degrees centered on the Text current drawing origin. The change to the orientation is permanent, when the Text object is rendered the new angle will be the reference for future rotation transforms.
Parameters:
degs: Number - The angle of rotation measured in degrees counter-clockwise for RHC coordinate grids and clockwise for SVG coordinate grids.
Syntax:
obj.scale(xScale[, yScale]);
Description:
Changes the dimensions of the Text by multiplying width by 'xScale' and height by 'yScale' (if specified). If 'yScale' is undefined then 'xScale' value is used to scale the both width and height. This scaling permanently changes the size of the Text as it will be rendered to the canvas. The scaling is centered on the drawing origin so the object doesn't shift position, just expands or contracts about the drawing origin.
If obj.lineWidthWC is defined then the width of any border will be scaled by 'xScale'. If the lineWidthWC is undefined then the line width used for borders will remain unaffected by this scale method.
Parameters:
xScale: Number - A number by which the object's X dimensions are scaled, negative numbers will flip the object horizontally if the num.
yScale: Number - (optional) A number by which the object's Y dimensions are scaled, negative numbers will flip the object vertically.
Syntax:
obj.skew(degH, degV);
Description:
Applies a 'hard' transform matrix to skew the text by an angle of 'degH' from the X axis, and by an angle of 'degV' from the Y axis.
Parameters:
degH: Number - Skew angle measured in degrees counter-clockwise from the X axis.
degH, degV: Numbers - Skew angle measured in degrees counter-clockwise from the Y axis.
Text objects like all objects and Group have a 'transform' property which consists of a matrix holding the geometric transforms to be applied to the object when it is rendered to the canvas. The transform matrix is built up by calling the obj.transform methods. These transforms do not affect the object definition, the transform matrix is reset to the identity matrix after the Text object is rendered. The supported methods are:
obj.transform.translate(xOfs, yOfs); obj.transform.scale(xScl, yScl); obj.transform.rotate(degs); obj.transform.skew(degH, degV); obj.transform.revolve(deg);
see obj.transform methods for details.
Syntax:
obj.enableDrag(grabCallback, dragCallback, dropCallback);
Description:
This method creates an object type Drag2D which is assigned to the obj.dragNdrop property. Drag2D objects encapsulate the event handlers that run when mousedown, mousemove and mouseup events occur within the bounding box the text as drawn on the canvas. The event handlers call the user defined callback functions passed to 'enableDrag'. 'grabCallback' is called on a mousedown events, 'dragCallback' and 'dropCallback' functions are called on subsequent mousemove and mouseup or mouseout events. The current cursor location is passed to the callback functions as an object with properties 'x' and 'y' holding the cursor coordinates measured in world coordinates. The obj will have drag and drop capability enabled whenever it is rendered to the canvas.
To simplify writing grab, drag and drop functions, the callbacks are executed in the scope of a Drag2D object which has properties to supply these values. All the handy properties in scope of the handlers are listed in the Drag2D section below.
When drag-n-drop is enabled on 'obj' the canvas mousedown event listener is armed to check for mousedown events within its outline. As long as the obj.dragNdrop property is not 'null', the mousedown listener is activated every time 'obj' is rendered to the canvas. The drag-n-drop is de-activated if the canvas is cleared by a call to cgo.clearCanvas, or by calling the obj.disableDrag method.
Parameters:
grabCallback:Function or null - This function to be called when a 'mousedown' event occurs within the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dragCallback:Function or null - This function to be called when a 'mousemove' event occurs after the mouse down event has occurred with the outline of 'obj'. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dropCallback:Function or null - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
Syntax:
obj.disableDrag();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of Obj2D to be checked for a hit on mousedown events.
Parameters:
none
ClipMask constructor
Syntax:
var obj = new ClipMask([data [, options]]);
Description:
This function returns a ClipMask object suitable for masking areas of the canvas where subsequent children of a Group will be rendered. The ClipMask object returned has all the hard and soft transform methods as Path or Shape objects. It doesn't have any properties such as fillColor or border etc. The outline should be closed, but if the 'Z' command is omitted from the outline definition it will be added automatically prior to rendering.
To apply a clip mask the ClipMask object must be a child of a Group, any Obj2D children of the Group that are drawn after the ClipMask is rendered will be clipped, only those parts of these objects lying within the clip mask outline will be drawn. The order of adding objects to a Group determines which child Obj2D (or child Group) will be clipped. Obj2D added to a Group before the ClipMask object are not clipped, those added after are clipped.
The canvas clipping path is automatically reset to the full canvas (ie. no clipping) by the render method after all children of the Group it is rendering are drawn. The clipping path may be manually reset by adding a blank ClipMask to the Group all objects added to the Group children after a blank ClipMask will not be clipped. A blank ClipMask is created by calling the ClipMask constructor with no path data parameter as follows:
var blankClip = new ClipMask();
Parameters:
data: String or Array - A string or array containing a series of commands and their associated coordinates defining the outline shape of the clipping mask. The full SVG set of commands is supported (see SVG / Cgo2D syntax). If the path data is undefined or an empty array then the ClipMask returned will represent the full canvas, ie. child objects of the group following the blank clip mask will not be clipped as they are rendered.
options: Object - This object may contain one key-value pair to pre-set the 'iso' property of the ClipMask object returned.
Example 1
Figure 1 shows an example of using a ClipMask. A Group is created comprising a red square SHAPE, a circular ClipMask and a blue triangle. The square is rendered first and is not clipped, the ClipMask circle is then rendered which sets up the clipping path and then the triangle is rendered. Only the part of the triangle within the clipping path is drawn. The clipping path will be reset to the full canvas (ie. no clipping) after the triangle is rendered.
A ClipMask example.
function testClip(cvsID) { var g, clipper = new ClipMask(shapeDefs.circle(50)), sqr = new Shape(shapeDefs.square(70), {fillColor:"red"}), tri = new Shape(shapeDefs.triangle(70), {fillColor:"blue"}), grp; g = new Cango(cvsID); g.setWorldCoordsRHC(-60, -60, 120); grp = new Group(sqr, clipper, tri); g.render(grp); }
Example 2
To make a ClipMask with a hole in it, create the larger ClipMask then create the hole as a Shape (or Path) object with the reverse winding to the ClipMask outline, then use the ClipMask's 'appendPath' method to append the hole outline. The following code creates a ClipMask in the shape of an annulus.
var clipper = new ClipMask(shapeDefs.circle(100)); var hole = new Shape(shapeDefs.circle(50)); hole.revWinding(); clipper.appendPath(hole); // clipper is now a annulus shaped mask
ClipMask properties
Property Name | Type | Range / Description |
---|---|---|
iso | Boolean or String | true, 'iso' or 'isotropic' will preserve the aspect ratio of a mask in non-isotropic coordinates. false will allow independent X and Y scaling. ClipMask default iso value is true. |
ClipMask methods
Syntax:
obj1.appendPath(obj2 [, delMove]);
Description:
appendPath extends the array of draw commands defining the outline of the ClipMask obj1 by appending the draw commands of obj2. If the optional 'delMove' parameter is 'true', then the initial 'moveTo' command is deleted from the array of commands to be appended.
Parameters:
obj2: Path (or Shape or ClipMask) object - The object whose drawCmd array elements are to be appended to the drawCmd array of obj1.
delMove: Boolean - If 'true' the initial 'moveTo' command of obj2 path outline is omitted. This results in the outline path of obj1 being joined to the outline of obj2. If 'delMove' evaluates to false, then the 'moveTo' command and its coordinates just become the next command in the outline of obj1 which means the mask outline will comprise two shapes.
Syntax:
obj.rotate(degs);
Description:
Rotates the ClipMask by 'degs' degrees centered on the current drawing origin. The change to the orientation of the outline is permanent, when the clip mask is applied the new angle will be the starting angle for any rotation transform applied prior to rendering.
Parameters:
degs: Number - The angle of rotation measured in degrees counter-clockwise for RHC coordinate grids and clockwise for SVG coordinate grids.
Syntax:
obj.scale(xScale[, yScale]);
Description:
Changes the dimensions of the ClipMask outline by multiplying X coordinates by 'xScale' and Y coordinates by 'yScale' (if specified). If 'yScale' is omitted or invalid then 'xScale' value is used to scale the Y coordinates. This scaling permanently changes the size of the clip mask as it will be applied by the 'render' method. The scaling is centered on the drawing origin.
Parameters:
xScale: Number - A number by which the object's X dimensions are scaled, negative numbers will flip the object horizontally if the num.
yScale: Number - (optional) A number by which the object's Y dimensions are scaled, negative numbers will flip the object vertically.
Syntax:
obj.translate(x, y);
Description:
Adds the values x and y to all draw commands coordinates of the ClipMask outline. Effectively making a permanent shift of the outline by x and y from the drawing origin. The change to the drawing origin is permanent. The center of subsequent rotation and scaling will be about this new drawing origin.
Parameters:
x, y:Numbers - X and Y offset of the object from its current drawing origin.
ClipMask objects like all Obj2D and Group have a 'transform' property which consists of a matrix holding the geometric transforms to be applied to the ClipMask when it is rendered to the canvas. The transform matrix is built up by calling the obj.transform methods. These transforms do not affect the object definition, the transform matrix is reset to the identity matrix after the ClipMask object is rendered. The supported methods are:
obj.transform.translate(xOfs, yOfs); obj.transform.scale(xScl, yScl); obj.transform.rotate(degs); obj.transform.skew(degH, degV); obj.transform.revolve(deg);
see obj.transform methods for details.
Groups
Cango provides the Group object to enable multiple Obj2D and Group to be treated as a single entity for transformations and rendering to the canvas. Since other Groups can be added to a group, it provides the mechanism for inheritance of any transforms applied to parent groups.
Group constructor
Syntax:
var obj = new Group(...);
Description:
Creates a Group object with a 'children' array property which hold Obj2D and other Groups. The Group object has methods to apply transforms to children, and recursively apply them to the children of child Groups. The objects forming the Group's children may be passed as parameters, either individual Obj2D or Groups or arrays of either. More objects can be added to the group after creation, by using the addObj method.
Parameters:
. . . :Objects, Group or arrays or either - The 'arguments' property is parsed to extract all Obj2D and Groups and they are added to the grp.children array in sequence left to right.
Group properties
Property Name | Type | Range / Description |
---|---|---|
children | Array | Holds the Groups's Obj2D or Group objects in the order in which they were added. |
Group methods
addObj
Syntax:
grp.addObj(...);
Description:
The method adds Obj2D or Group to grp. The arguments can be single Obj2D or Group or arrays of either. The array structure is discarded and the individual Obj2D or Group are added to the grp.children array.
Parameters:
. . . : Path, Shape, Img, Text, ClipMask, Group objects or arrays of these - The 'arguments' property is parsed to extract all Obj2D and Group and they are added to the grp.children array.
deleteObj
Syntax:
grp.deleteObj(obj);
Description:
If 'obj' is a child of the Group 'grp' then it is removed from the array of children.
Parameters:
obj: Obj2D or Group - The child to be removed from the Group.
Syntax:
grp.rotate(degs);
Description:
Applies a rotation matrix to the 'hard' transform matrix of all the children of the Group, 'grp' recursively, effectively rotating all descendent objects by 'degs' degrees centered on each Obj2D's drawing origin. This is a 'hard' transform, the orientation of the Obj2D becomes the reference orientation for subsequent transforms and rendering.
Parameters:
degs: Number - The angle of rotation measured in degrees positive anti-clockwise in RHC coordinate grids and clockwise in SVG coordinate grids.
Syntax:
grp.scale(xScale[, yScale]);
Description:
Applies a scale transform to the 'hard' transform matrix of the Group 'grp' recursively, effectively scaling the width or all X coordinates by 'xScale' and multiplying the height or Y path coordinates by 'yScale'. If yScale is undefined yScale is set equal to xScale so the scaling is isotropic. This scaling permanently changes the size of the group's child Obj2D.
Note scaling by (-1, 1) flips the group's descendent Obj2D horizontally and scaling by (1, -1) flips the objects vertically.
Parameters:
xScale: Number - A number by which descendent Obj2D X dimensions are scaled, negative numbers will flip the objects horizontally if the num.
yScale: Number - (optional) A number by which descendent Obj2D Y dimensions are scaled, negative numbers will flip the objects vertically.
Syntax:
grp.skew(degH, degV);
Description:
Applies a skew transform to the 'hard' transform matrix of the Group 'grp' recursively, effectively skewing all the descendent objects by an angle of 'degH' from the X axis, and by an angle of 'degV' from the Y axis.
Parameters:
degH: Number - Horizontal skew angle measured in degrees counter-clockwise for RHC grids and clockwise for SVG grids.
degH, degV: Numbers - Vertical skew angle measured in degrees counter-clockwise for RHC grids and clockwise for SVG grids.
Syntax:
grp.translate(x, y);
Description:
Applies a translate transform to the 'hard' transform matrix of the Group recursively, effectively making a permanent shift of each descendent child Obj2D by x and y from their drawing origins. This is a 'hard' transform the change relative to the drawing origin becomes the reference position for future transform or rendering. The center of rotation and scaling will be this new drawing origin.
Parameters:
x, y: Numbers - X and Y offsets of the child Obj2D from their current drawing origin.
Groups have a 'transform' property which consists of a matrix holding the geometric transforms to be applied recursively to the child Obj2D of the group when they are rendered to the canvas. The transform matrix is built up by calling the grp.transform methods. These transforms do not change the child Obj2D definitions, the transform matrix is reset to the identity matrix after the descendent child Obj2D are rendered. The supported methods are:
grp.transform.translate(xOfs, yOfs); grp.transform.scale(xScl, yScl); grp.transform.rotate(degs); grp.transform.skew(degH, degV); grp.transform.revolve(deg);
see transform methods for details.
Syntax:
grp.enableDrag(grabCallback, dragCallback, dropCallback);
Description:
Calls the enableDrag method on all grp's children recursively. The three user defined call back functions 'grabCallback', 'dragCallback', 'dropCallback' are passed to each child's 'enableDrag' method.
Parameters:
grabCallback:Function or null - This function to be called when a 'mousedown' event occurs within the outline of the grp's descendent object. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dragCallback:Function or null - This function to be called when a 'mousemove' event occurs after the mouse down event has occurred with the outline of the grp's descendent object. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
dropCallback:Function or null - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An object containing the current cursor 'x' and 'y' world coordinate position as properties is the only parameter passed when the function is called.
Syntax:
grp.disableDrag();
Description:
Sets the grp.dragNdrop property to 'null' and removes the reference to grp's children from the array of Obj2D to be checked for a hit on mousedown events.
Parameters:
none
Soft Transforms
All Obj2D and Groups have a transform property which consists of a matrix holding the geometric transforms to be applied to the object when it is rendered to the canvas. The transform matrix is built up by successive calls to the transform methods. These transforms do not affect the object definition, the transform matrix is reset to the identity matrix after the Obj2D are rendered.
When a soft transform is applied to a Group it will be inherited by all the Group's children. Child Obj2D or Groups may have transform methods applied to them directly, these are combined with the inherited transforms and the resulting net transform propagated to any descendent Obj2D.
Note that transform.rotate, transform.skew and transform.scale operations are always referenced to the Obj2D's drawing origin regardless of any obj.translate operations which may move the object's drawing origin with respect to the world coordinate origin. The transform.translate and transform.revolve are always referenced to the world coordinate drawing origin.
transform methods
Syntax:
a.transform.revolve(deg);
Description:
Applies a transform to the 'soft' transform matrix which revolves the Obj2D by 'degs' degrees centered on the world coordinate origin (not the object's drawing origin). If no transform.translate has been applied prior to this method being called, transform.revolve behaves identically to transform.rotate, since the object drawing origin has not been moved from the world coordinate origin, but if the object has been translated, this method revolves the object around the world coordinate origin now some distance from the object.
When applied to a Group this transform matrix is applied recursively to all the descendent Obj2D of the Group.
Parameters:
deg: Number - The angle of revolution measured in degrees +ve anti-clockwise.
Syntax:
a.transform.rotate(deg);
Description:
Applies a transform to the 'soft' transform matrix which rotates the Obj2D by 'deg' degrees centered on the object's drawing origin. This rotation is independent of any transform.translate calls that may have moved the drawing origin from the world coordinate origin.
When applied to a Group this transform matrix is applied recursively to all the descendent Obj2D of the Group.
Parameters:
deg: Number - The angle of rotation measured in degrees +ve anti-clockwise.
Syntax:
a.transform.scale(xScale[, yScale]);
Description:
Applies a transform to the 'soft' transform matrix which scales the X dimensions of the Obj2D or descendent Obj2D in the case of Group, by a factor 'xScale' and if yScale is defined, scales the Y dimensions by yScale. If yScale is undefined then the Y dimensions are scaled by xScale ie. isotropic scaling. The object's dimensions enlarge or contract relative to the object's drawing origin, independent of any transform.translate that may have been applied.
When applied to a Group this transform matrix is applied recursively to all the descendent child Objects of the Group.
Parameters:
xScale: Number - A number >0 by which the objects dimensions relative to it's drawing origin are scaled.
yScale: Number - (optional) A number >0 by which the objects dimensions relative to it's drawing origin are scaled.
Syntax:
obj.transform.skew(degH, degV);
Description:
Applies a transform to the 'soft' transform matrix which distorts the shape of the Obj2D by offsetting the outline X coordinates progressively up to a value of 'degH', and progressively offsetting the Y coordinates up to a value of 'degV'.
When applied to a Group this transform matrix is applied recursively to all the descendent Obj2D of the Group.
Parameters:
degH: Number - Skew angle measured in degrees counter-clockwise from the X axis.
degH, degV: Numbers - Skew angle measured in degrees counter-clockwise from the Y axis.
Syntax:
a.transform.translate(x, y);
Description:
Applies a transform to the 'soft' transform matrix which effectively adds an offset of 'x' to the X coordinates and an offset of 'y' to the Y coordinates of the of the Obj2D. The center of rotation and scaling will be not be affected by this translation of the object's drawing origin, but revolve transform is affected.
When applied to a Group this transform matrix is applied recursively to all the descendent child Obj2D of the Group.
Parameters:
x, y: Number - The distance in the X and Y directions respectively that the object will be moved relative to the world coordinate origin.
Rendering
Cango has only one methods to actually draw objects onto the canvas: cgo.render.
render takes a Group (or a single Obj2D) and renders it to the canvas. In the case of Group, the entire family of descendant Obj2D is rendered. All transforms that have been accumulated by any of the Group or Obj2D in the family tree are applied. The Group transforms are propagated down the family tree structure to determine the final positions of the Obj2D prior to rendering. render takes a second optional 'clear' parameter which, if it evaluates to true will cause the canvas to be cleared of all previous drawing prior to rendering the new family tree of objects.
After an Obj2D is rendered the 'soft' transform matrix applied to the Obj2D is reset to the identity matrix i.e. no transforms. After a Group is rendered the 'soft' transform matrix for the whole family tree are reset recursively. This means all animation pathFn functions should re-apply any 'soft' transforms prior to each frame.
After all the children of a Group have been rendered any clipMasks that has been applied will be reset to the full canvas. This means that rendering a clipMask on its own will have no effect, it will be applied and then cleared within the same render call. ClipMasks are only effective within a Group and will only clip those Objects added to the Group after the clipMask.
Cango method details
Syntax:
cgo.render(obj, clear);
Description:
Renders an Obj2D or the descendant Obj2D of the Group onto the canvas. The render method applies all the accumulated 'soft' transforms to each Obj2D as it is rendered.
Parameters:
obj: Obj2D or Group - The Obj2D or root Group of a family tree of objects to be rendered to the canvas.
clear: Boolean - If 'clear' evaluates to 'true', the canvas is cleared before rendering the Obj2D, if it evaluates to 'false', the canvas is not cleared and the Obj2D are added to any existing drawing.
Animation
Animations may be simply created by calling the Cango animation method. The calling syntax is as follow:
cgo.animation(initFn, drawFn, pathFn, options);
The 'initFn', is a user defined function that can apply transforms to the animated object prior to the animation commencing, the draw function 'drawFn' is then called to render the objects in their in this initial state. The 'drawFn' is function that actually does the rendering of the scene at each frame. The 'pathFn' is a function called at every frame to calculate the new positions, rotations etc. and apply the corresponding transforms to the objects forming the animation. The 'options' parameter is an object which can hold any user defined properties that may be required by the initFn, drawFn or pathFn.
All animations on a canvas are controlled by a master timeline with all animated objects transformed and rendered at each frame even if they are drawn on different layers. The 'pathFn' functions of all animated objects are passed the elapsed time along the same timeline at each frame ensuring the motion of different objects are synchronized. The animation control methods are:
The animation frame rate is set by the 'requestAnimationFrame' utility, the time between frames will not be constant but will generally be around 17 msec (~60 frames/second).
The animation control methods cgo.playAnimation, cgo.pauseAnimation, cgo.stepAnimation, cgo.stopAnimation. These methods may be called on any Cango context from the background canvas or any layer, they all refer to the same methods. The 'playAnimation' and 'stepAnimation' methods follow the same sequence for each frame for each animated object on all layers:
- Clear the canvas on which each animation's calling Cango context is defined.
- Call the animation's 'pathFn', passing the elapsed time along the timeline for this frame and the 'options' object.
- Call the animation's 'drawFn' to render the Obj2D or Group's descendent Obj2D onto the canvas. Rendering resets all the soft transforms and resets any clip mask to the full canvas.
- Swap the animation's nextState and currState objects.
- Save the time at which the frame was drawn in the 'currState.time' property of each animation.
Cango Animation methods
Syntax:
animId = cgo.animation(initFn, drawFn, pathFn, options);
Description:
The animation method creates an AnimObj object with properties that may be useful to the initFn, drawFn and pathFn functions which are called in the scope of this AnimObj object.
The initFn is called immediately, it is designed to apply transforms to the various objects setting up the initial state (prior to the animation starting). The 'drawFn' is then called to render the scene in this initial state. Once the animation has been started, the pathFn is called at each frame along the timeline. The pathFn calculates the new movement transforms and applies them to objects. The 'drawFn' is then called to render the frame.
Parameters:
initFn: Function - A function to be called to initialize or create additional properties of nextState (if required) and apply these transforms to the object. When called initFn is passed one parameter, the 'options' object.
drawFn: Function - A function to be called after 'initFn' and 'pathFn' calls to actually renders the animated objects to the canvas. When called drawFn is passed one parameter, the 'options' object.
pathFn: Function - A function to be called to update the object properties to the values to be used when drawing the next frame. When called pathFn is passed two parameters 'time' and the 'options' object. The 'time' will be in 'msecs' and represents the elapsed time along the timeline, the same format as 'this.currState.time so that the elapsed time since the last frame was drawn will be:
var dt = time - this.currState.time; // in milliseconds
options: Object - An object storing any variables or constants that may be required by the initFn, drawFn or pathFn. The options object is passed as an argument to these functions.
Syntax:
cgo.deleteAnimation(animId);
Description:
All animations are paused and the animation whose ID is 'animId' is deleted. This ID value was returned when the animation was created by a call to 'animation' method. The animation's AnimObj is removed from the current array of animations. The frame currently drawn on the canvas will remain frozen. This method may be called on any Cango context on any layer in the stack and will still delete the nominated animation.
Parameters:
animId: String - An ID string returned when the animation was created.
Syntax:
cgo.deleteAllAnimations();
Description:
Deletes all the animations from the background layer and from any and all canvas layers. All the animations are stopped and animation definitions removed from the current array of animations, all the animated objects will remain frozen where they were last drawn. This method may be called on any Cango context on any layer and will still clear all animations on all layers.
Parameters:
none.
Syntax:
cgo.playAnimation([startTime[, stopTime]]);
Description:
Starts all animations that have been defined for Cango contexts on this canvas or stack of canvases (if overlay canvases have been created). At each frame the elapsed time along the timeline is passed to the pathFn of all animated objects so they can apply the movement transforms. By default, the timeline is traversed from time 0, but if a 'startTime' is passed then this will be the time initially passed to the pathFn. The animation timeline is traversed by drawing a frame and then making a call to 'requestAnimationFrame' to draw the next and so on until the 'stopAnimation' method is called or, if a 'stopTime' was passed then the animation will stop as soon as the elapsed time since starting equals or exceeds this stopTime.
Parameters:
startTime: Number - Optional time in milliseconds along the timeline where the animation playing will commence.
stopTime: Number - Optional time in milliseconds along the timeline where the animation will cease and the mode will be set to 'stopped'.
Syntax:
cgo.pauseAnimation();
Description:
Stops any current animation and saves the elapsed time since the start of the animation. When 'playAnimation' or 'stepAnimation' are called the animation will resume from the current time offset from the start. This differs from the 'stopAnimation' call which forces the animation to return to the start when 'playAnimation' or 'stepAnimation' is called. The context 'cgo' may be attached to the background or any overlay canvas and will have the same effect.
Parameters:
none.
Syntax:
cgo.stepAnimation();
Description:
If the animation is currently playing this call does nothing. If paused or stopped the animation will request a frame to be drawn on the background canvas and all overlay layers with the elapsed time of the animation advanced by the current value of 'stepTime'. One frame will be drawn and the animation put into the 'paused' state. The value of 'stepTime' may be set by setPropertyDefault, the default value is 50 msec. The context 'cgo' may be attached to the background or any overlay canvas and will have the same effect.
Parameters:
none.
Syntax:
cgo.stopAnimation();
Description:
Stops any current animation and reset the elapsed time into the animation back to 0. When 'playAnimation' or 'stepAnimation' is subsequently called the animation will resume from the beginning of the animation timeline. This differs from the 'pauseAnimation' call which restart the animation from the current elapsed time along the animation timeline. The context 'cgo' may be attached to the background or any overlay canvas and will have the same effect.
Parameters:
none
AnimObj object
To implement this animation model, the Cango animation method creates a object of type AnimObj which encapsulates references to the Cango context that created the animation and other properties that may be useful to the 'initFn', 'drawFn' and 'pathFn'. These functions are called in the scope of the AnimObj object ie. within these functions 'this' will refer to the AnimObj object so all its properties are readily available.
The 'pathFn' may be of a type that needs to refer to the values used to drawn the frame currently on the screen and the time it was drawn, to calculate values for the next frame. To assist in such situations, Cango animation provides the currState and nextState objects. All the newly calculated values that the pathFn will need to create the transforms for the next frame should be stored in the object this.nextState. After playAnimation or stepAnimation renders the object to the canvas it swaps this.currState and this.nextState objects so that the 'as rendered' properties are always available in this.currState. The pathFn should treat this.currState properties as 'read-only' using the values to make calculations, write these to this.nextState then apply the transforms to the object.
AnimObj properties
Property | Type | Description |
---|---|---|
gc | Cango object | The graphics context that will render the object onto the canvas at each frame along the timeline. The pathFn can make use of this graphics context to call Cango methods such as setPropertyDefault etc. |
nextState | Object | A JavaScript object provided to hold all the user defined properties that will be changed at for each frame. The 'pathFn' updates the nextState property values prior to 'obj' being rendered. Once the frame is rendered 'nextState' is swapped with the 'currState' object so the 'as rendered' values of the property values are available to the pathFn as a reference when next called. The pathFn will then overwrite the nextState values with new values for the next frame and so on. |
currState | Object | A JavaScript object to hold a copy of the 'nextState'. This object should be considered read-only as it will be swapped with the 'nextState' object after each frame is rendered. The currState object will always have the property 'time' which holds the time (in msec) at which the frame on the canvas was rendered. All other properties will be user defined 'nextState' properties. |
options | Object | An object that is available to the 'initFn', 'drawFn' and 'pathFn' to hold values useful in setup, drawing or generating the 'nextState'. If the 'pathFn' calls a Tweener.getVal method the 'options' object can be used to hold the array of keyframe values to be interpolated. |
Animation example
Here is the source code for an animation which rotates a Text object through 360 degrees then back again at the same time scaling the object to twice its size and back again. The animation starts after a 1 sec delay then repeats the movement and the delay period indefinitely.
Figure 2. Text object with animated transform.translate and transform.scale.
function orbitDemo(cvsID) { var txt = new Text("Hullo", { fillColor:"blue", fontSize:8, lorg:5}), orbitData = {radius:60, va:1.5, ang:0}; function initTxt(opts) { txt.transform.translate(opts.radius, 0); } function drawTxt(opts) { this.gc.render(txt); } function orbit(time, opts) { var dt = time - this.currState.time; // time since last frame opts.ang += opts.va*dt/1000; // constant angular velocity if (opts.ang > 2*Math.PI) // wraparound for angle { opts.ang -= 2*Math.PI; } txt.transform.translate(opts.radius*Math.cos(opts.ang), opts.radius*Math.sin(opts.ang)); txt.transform.scale(2.5+ Math.cos(opts.ang)); } ballGC = new Cango(cvsID); ballGC.setWorldCoordsRHC(-100, -100, 200); ballGC.animation(initTxt, drawTxt, orbit, orbitData); ballGC.playAnimation(); }
Tweener interpolation utility
When writing a Cango animation 'pathFn' functions, the aim is to generate values of position, scale, rotation etc or some style property that can be set for an object being animated. New values need to be generated for each frame so the 'pathFn' is called prior to each frame. If the property is specified as an array of key values and key times then the next frame values can be obtained by interpolating between these keyframe values. The Tweener object is provided to simplify this task. A Tweener holds the basic parameters of a timeline and has just one method: getVal which will do the interpolation calculations based on key frame values and the time along the timeline.
Tweener constructor
Syntax:
var twnr = new Tweener(delay, dur, loopStr);
Description:
This utility is provided to simplify interpolation between elements in an array of key values. Creating a Tweener object sets up a timeline of length 'dur' milliseconds which optional 'delay' to the start of the animation and whether the animation repeats specified by the 'loop' parameter.
Parameters:
delay:Number - A time in milliseconds that must be exceeded before interpolating key values begins. When a Cango animation is started by a call to the 'playAnimation' method times starting at 0 msec are passed to path functions.
dur:Number - The duration of the animation starting after 'delay' milliseconds and lasting 'dur' milliseconds. Interpolated values will be returned for time between delay msec and delay+dur msec.
loopStr:String - The 'loopStr' parameter can take two values that will cause animation looping: 'loop' and 'loopAll'. After the initial delay (if delay is non-zero) 'loop' will cause the animated sequence that is 'dur' msec long to be repeated without repeating the delay. 'loopAll' will cause the delay and the animation to repeat, so the repeat interval will be delay+dur msec long. Either value will repeat its sequence indefinitely or until 'stopAnimation' or 'pauseAnimation' stops more calls being made to animation path functions. If the delay = 0 there is no difference in behaviour between 'loop' and 'loopAll'.
Tweener methods
Syntax:
var val = twnr.getVal(time, keyValues[, keyTimes]);
Description:
The getVal method is designed to be used in an animation pathFn which will be called immediately prior to each animation frame being rendered. It returns a value for some property at time 'time' along a timeline of duration twnr.dur by interpolating between elements in an array of key values. The keyframe values are specified in the keyValues array. It is assumed that the first key value represents the property at the beginning of the Tweener timeline 'dur' period and the last key value is the value at the end of 'dur' period.
The key frame times corresponding to the key values may be passed to 'getVal' in a separate keyTimes array. If no 'keyTimes' array is passed to the 'getVal' method then the key values are assumed to be equally spaced over the 'dur' time.
Parameters:
time: Number - Elapsed time along the Tweener timeline, measured in milliseconds when getVal is called. The value starts at 0 and continues incrementing until Cango.stopAnimation is called, which will reset the elapsed time to 0. The 'getVal' method will not start interpolating until the time exceeds the Tweener 'delay' time. It then commences interpolating the value of the properties based on the time elapsed since starting as a percentage of the 'duration' parameter. The animation will last for 'dur' milliseconds. It will continue to return the last keyValue for all times exceeding delay+dur unless the timeline has looping enabled.
keyValues: Number or Array of Numbers - A single number will represent the static value for the entire animation. If 'keyValues' is an array then its elements represent the key values in the animation. If the array of values has 2 or more elements, the first will be the initial value returned after time 'delay' milliseconds. The last value will be the value at the finish of the animation after time interval 'delay+dur'.
keyTimes: Array of Numbers - An array holding the keyframe times corresponding to the keyValues. The keyTimes array must have the same number of elements as its matching values array. Time values are specified as a percentage of the Tweener.dur property so the values are limited to the range 0 to 100. If keyTimes is undefined then the key values are assumed to be equally spaced over the 'dur' time.
Simple Tweener example
Here is the source code for an animation which changes the fillColor a circle from red to green then blue then back to red. The animation lasts 3 seconds and repeats the cycle indefinitely.
Figure 3. Shape object with animated "fillColor" property.
function animColor(cvsID) { var g, disc = new Shape(shapeDefs.circle(50)), colData = { r:[255, 0, 0, 255], g:[0, 200, 0, 0], b:[0, 0, 255, 0] }, colTwnr = new Tweener(0, 3000, "loop"); function drawDisc(opts) { this.gc.render(disc); } function pathBall(time, opts) { var rVal = Math.round(colTwnr.getVal(time, opts.r)), gVal = Math.round(colTwnr.getVal(time, opts.g)), bVal = Math.round(colTwnr.getVal(time, opts.b)); disc.setProperty("fillColor", "rgb("+rVal+","+gVal+","+bVal+")"); } g = new Cango(cvsID); g.setWorldCoordsRHC(-50, -50, 100); g.animation(null, drawDisc, pathBall, colData); g.playAnimation(); }
Drag-and-Drop
Drag-n-drop can be enabled on any Obj2D or Group by calling the obj.enableDrag method passing as parameters references to the event handlers to be called when mousedown, mousemove and mouseup events occur. Drag-and-Drop can be disabled by calling the obj.disableDrag or by clearing the canvas on which the object was drawn.
Drag2D Object
The 'enableDrag' method creates a object type Drag2D which is assigned to the obj.dragNdrop property. Drag2D objects encapsulate the event handlers that run when mousedown, mousemove and mouseup events occur within the outline of the Obj2D as drawn on the canvas. When a mousedown event occurs the handler enables listening for mousemove, mouseup and mouseout event, it then updates the values the Drag2D properties and calls the user defined 'grabHandler' callback. Subsequent mousemove events will similarly update the Drag2D properties and call the user defined 'dragHandler'. The 'dropHandler' callback is called when either a mouseup event occurs or the cursor moves off the canvas generating a mouseout event. The callbacks are passed the current position of the cursor on the canvas in world coordinates. The callback functions are executed in the scope of the obj.dragNdrop object.
Enabling drag-n-drop on a Group recursively enables the drag-n-drop on all the group's descendent Obj2D. If a child Obj2D has drag-n-drop enabled this handler will take precedence over any parent Group drag-n-drop handler. So an Obj2D which is a child of a Group will move when any child of the group is dragged but will also move independent of the group if it has drag-and-drop independently enabled.
Drag2D properties
The obj.dragNdrop is a Drag2D object which has as properties several variables often required when writing event handlers, such as 'grabOfs', the X and Y offset of the cursor from the drawing origin of the Obj2D when the mousedown event occurred. Since the callbacks are executed in the scope of the obj's dragNdrop object, 'this' will refer to the Drag2D object so its properties listed below will be available:
Property | Type | Description |
---|---|---|
cgo | Cango object | This makes the Cango methods such as this.cgo.clearCanvas() readily accessible from the scope of the Drag2D object. |
target | Group or Obj2D | Group or Obj2D actually being dragged, the Group or Obj2D whose 'enableDrag' method was called. |
dwgOrg | Object | Object with 'x' and 'y' properties holding the 'as rendered' coordinates of the drawing origin (measured in world coordinates) of the target Obj2D or Group. |
grabOfs | Object | Object with properties x, y holding the offset of the cursor coordinates from the target drawing origin at the time of the mousedown (grab) event, measured in world coordinates. |
grabCsrPos | Object | Object with properties x, y holding the cursor coordinates saved at grab time, measured in world coordinates. |
Example
As an example of the drag-n-drop method, here is a code snippet that simply moves an object with the cursor:
Figure 4. Simple drag and drop example.
function dragBox(cvsID) { var g, boxOutline = ['M', 0, 0, 'l', 40, 0, 0, 100, -40, 0, 'z'], box = new Shape(boxOutline, { fillColor:'orange', border:true, strokeColor:"brown"}); function dragObj(mousePos) { var wPos = {x:mousePos.x - this.grabOfs.x, y:mousePos.y - this.grabOfs.y}; this.target.transform.translate(wPos.x, wPos.y); g.render(this.target, true); // true => clear canvas }; g = new Cango(cvsID); g.setWorldCoordsRHC(-100, -100, 400); box.enableDrag(null, dragObj, null); g.render(box); }
SVG and Cgo2D data syntax
The outlines for Path, Shape and ClipMask objects can be defined by a string containing SVG path data format or an array containing Cgo2D data format. Both these format use the same syntax which comprises single letter commands such as 'M' for move, 'L' for a line segment, 'C' for a cubic Bezier curve, etc and 'Z' for a close path instruction. the commands are followed by their associated coordinates.
Commands are case sensitive, uppercase command coordinates are interpreted as absolute i.e. measured from the world coordinate origin (0,0). Lowercase command coordinates are interpreted as being relative to the current pen position i.e. the end point of previous command. The SVG standard requires the first command must always be a move command ('M' or 'm') followed by the x, y values of the starting point of the path in world coordinates. This is followed by an arbitrarily long set of commands and their associated parameters and coordinates.
SVG data is always in a string and the coordinates are interpreted as Left Handed Cartesian Y coordinate values increase up the canvas and angles increase clockwise. Cgo2D array data are interpreted as Right Handed Cartesian, so Y values increase down the canvas and angles increase counter-clockwise.
SVG / Cgo2D syntax details
Command |
Parameters |
Description |
---|---|---|
M |
x,y |
moveto: Moves the pen to a new location. No line is drawn. All path data must begin with a 'moveto' command. |
Line Commands |
||
L |
x,y |
lineto: Draws a line from the current point to the point (x,y). |
H |
x |
horizontal lineto: Draws a horizontal line from the current point to x. |
V |
y |
vertical lineto: Draws a vertical line from the current point to y. |
Cubic Bezier Curve Commands |
||
C |
x1 y1 x2 y2 x y |
curveto: Draw a cubic Bezier curve to the point (x,y) where the points (x1,y1) and (x2,y2) are the start and end control points, respectively. |
S |
x2 y2 x y |
shorthand/smooth curveto: Draw a curve to the point (x,y) where the point (x2,y2) is the end control point and the start control point is the reflection of the last point's end control point. |
Quadratic Bezier Curve Commands |
||
Q |
x1 y1 x y |
quadratic Bezier curveto: Draw a quadratic Bezier between the last point and point (x,y) using the point (x1,y1) as the control point. |
T |
x y |
shorthand/smooth quadratic Bezier curveto: Draw a quadratic Bezier between the last point and point (x,y) using the reflection of the last control point as the control point. |
Elliptical Arc Curve Commands |
||
A |
rx, ry, x-rotation, large-arc-flag, sweep-flag, x, y |
elliptical arc: Draws and arc starting from the current point and ending at (x, y). The ellipse has the two radii (rx, ry). The x-axis of the ellipse is rotated by 'x-axis-rotation' relative to the x-axis of the world coordinate system. The 'large-arc-flag' and the 'sweep-flag' together define which way the arc joins to start and end point.
Cgo2D interprets the 'x-axis-rotation' coordinate is positive anti-clockwise and 'sweep-flag = 1' is interpreted as the arc sweeping in an anti-clockwise direction. SVG interprets the 'x-axis-rotation' as positive clockwise and 'sweep-flag = 1' flag is interpreted as the arc sweeping in a clockwise direction. |
End Path Commands |
||
Z |
- |
closepath: Closes the path. A line is drawn from the last point to the first. |
Relative coordinates:
To use path end point and control point coordinates relative to the last pen position use a lowercase letter for the command, eg. 'a' rather than 'A'.
As an example, here is the array of commands to draw a unit square with the drawing origin (0,0) at its center.
var cgoSquare = ['M', 0.5,-0.5, 'l',0,1, -1,0, 0,-1, 'z']; var svgSquare = "M 0.5 -0.5 l 0 1 -1 0 0 -1 z";
svgToCgo2D utility
SVG path data is often imported as a string copied from an SVG editor such as Inkscape. SVG path data will be designed for Left Handed Cartesian coordinates which have their Y coordinate values increase DOWN the canvas and the "A" segment arcs sweep clockwise. If this is to be used with Right Handed Cartesian coordinates the drawing will appear upside-down. The svgToCgo2D utility to provided to convert the SVG coordinates to the Cango world coordinates by flipping the sign of all Y coordinates and flipping and arc x-axis-rotation angle and sweep direction. SVG editors usually have the drawing origin for PATH data at the top left of the edit screen which introduces a large offset in all coordinates. The parameters xOfs, yOfs are added to the SVG coordinates to correct this, moving the path's drawing origin to a more useful position. The xOfs and yOfs are added prior to flipping the Y coordinate values.
var cgoPathdata = svgToCgo2D(svgPathStr [, xOfs, yOfs]);
Numerical path data
Cgo2D syntax allows a concession to the SVG command format, by accepting an array of data values with no command strings as a valid path definition. In such a case the initial 'M' command to move to the location of the first x,y pair is assumed, and the succeeding pairs are assumed to be preceded by an 'L' command. An example of storing coordinates values in this format:
var i, data = []; for (i=0; i<n; i++) { data.push(xFn(i), yFn(i)); // xFn producing x values, yFn producing y values } g.drawPath(data); // draw line starting at data[0],data[1] then n straight line segments
lorg (locate origin)
Location of the drawing origin within the bounding box of a Text or Img object is set by the parameter 'lorg'. The 'lorg' property can take integer values 1..9, lorg>=1 locates the top,left of the text box or image at the (x, y) position, lorg=2 locates the top, center of the box at the (x, y) position, and so on. Fig 5 shows the effect of each 'lorg' value on where a text object is drawn. Each text object in the figure is drawn at the x,y coordinates of the adjacent grid intersection, the text string itself specifies which lorg value was used when drawing the text.
Figure 5. Examples of the 9 possible values of lorg. Each label states its lorg value. Each label is drawn at the coordinates of its nearest grid intersection. The red labels demonstrate that the lorg position is also the center of rotation.
Colors:
Fill color or stroke color parameters may be specified either by a reference to a canvasGradient object or by a string specifying the a color in one of the CSS color formats.
CSS Color formats
There are five different formats that can be used to define a color for use in the canvas:
- 6 Digit RGB hex notation, '#rrggbb', where 'rr' sets the Red level (00 to ff), 'gg' sets the Green and 'bb' sets the Blue level.
- 3 Digit RGB hex notation '#rgb', this is converted into six-digit form (#rrggbb) by replicating digits, not by adding zeros. For example, #fb0 expands to #ffbb00.
- RGBA notation 'rgba(r, g, b, a)' where r, g and b are decimal numbers in the range 0 to 255 representing the Red, Green and Blue levels respectively and a is the Alpha or transparency value, 0 being fully transparent and 1.0 fully opaque.
- RGB notation "rgb(r, g, b)" where r, g and b are decimal numbers in the range 0 to 255 representing the red, green and blue levels respectively. This is a suitable format for entering dynamic color values. The calculated r, g and b values may be passed to drawing methods by the string 'rgb('+r+','+g+','+b+')'.
- Predefined colors names specified by the Extended CSS Color List eg. 'red', 'blue', 'maroon', 'palegoldenrod', 'wheat' etc. There are 143 standard colors named in the list.
LinearGradient and RadialGradient Objects
The LinearGradient and RadialGradient objects encapsulates the instructions from making canvasGradient objects just as if ctx.createLinearGradient or ctx.createRadialGradient had been called. The difference is that they expect the coordinates passed as parameters to be in world coordinates not pixels. If the 'fillColor' property of an object is assigned to be a LinearGradient or RadialGradient object then the coordinates defining the gradient are relative to the drawing origin of the object, so if the object is translated then the gradient fill will move with the object. The LinearGradient fill will also scale and rotate with the object
The LinearGradient and RadialGradient constructors are global and are independent of any particular Cango instance.
LinearGradient or RadialGradient objects may be used as the fill color for cgo.clearCanvas methods. The world coordinates passed to the constructor are relative to the current world coordinate drawing origin 0,0.
Example 1:
An example of the LinearGradient is:
var gradObj = new LinearGradient(-2,2, 2,-2); gradObj.addColorStop(0, 'yellow'); gradObj.addColorStop(0.5, 'green'); gradObj.addColorStop(1, 'black'); g.setWorldCoordsRHC(-2, -2, 4); // 4x4 square, 0,0 in center g.drawShape(shapeDefs.square(4), {fillColor:gradObj});
Example 2:
An example of the RadialGradient is :
var grad = new RadialGradient(0, 0, .5, 1, 1, 2.5); grad.addColorStop(0, '#aabbff'); grad.addColorStop(1, '#00f'); g.setWorldCoordsRHC(-2, -2, 4); // 4x4 square, 0,0 in center g.drawShape(shapeDefs.square(4), {fillColor:grad});
.
Zoom and Pan Utility
Support for zoom and pan of Cango drawings is provided by the global function initZoomPan. This function should be called after any canvas drawing layers have been created ensuring that the zoom and pan controls will be on the top layer of the stack and thus giving priority to then for any mouse click events. The function draws a semi-transparent set of controls in the top right corner of the canvas. When these control arrows are clicked the Cango contexts nominated will have their world coordinates modified to zoom or pan the canvas drawing.
Syntax:
initZoomPan(zpLayerId, gc, reDraw);
Description:
This function creates an overlay canvas and draws Zoom and Pan controls in the top right corner of a canvas element with ID 'zpLayerId'. Any or all the Cango contexts defined on any of the canvases in the stack can be zoomed and panned by clicking on these controls. The Cango contexts to be affected by zooming and panning are set by the 'gc' parameter. 'gc' may be a single Cango context or an array of contexts. Clicking the zoom controls will re-scaling the world coordinates of these contexts by 20% per click. Clicking the pan controls will add or subtract 50 pixels per click to the corresponding coordinate offset. When the scaling or position offsets have been applied, the Cango drawings on the canvas must be re-drawn to show the effect. The callback function to do this re-draw is also passed as the 'reDraw' argument.
Parameters:
zpLayerId:String - This is the string ID of the canvas layer that will hold the zoom and pan control buttons. This is the ID returned from a call to Cango.createLayer() method, so a call to this method may be used in place of the string.
gc:Cango context or Array of Cango contexts - This is the Cango graphics context that will have all its drawing zoomed or panned. If several Contexts are present on the background canvas or any canvas layer they may be passed as an array of Cango contexts, all will be zoomed or panned together as the control buttons are clicked.
reDraw:Function - The user defined function 'redraw' will be called to redraw all the objects on all the canvases in their new zoomed or panned size or position.
Example:
Figure 6. Example of zoom and pan controls, click on the buttons in the top right corner to zoom or pan the drawing. Clicking on the 'X' button returns the drawing to its original size and position.
The code for the zoom and pan example in Fig. 6 is shown below. Note that the line width of the Img border is set in world coordinates using the 'lineWidthWC' property so the border scales with zooming, whereas the border width of the yellow shape is set in pixels using the 'lineWidth' property and so doesn't scale with zooming.
function zoomPanTest(cvsID) { 'use strict'; var g, gL1, xmin = -300, ymin = -200, xspan = 750, Arc = "M0,50 h-150 a150,150 0 1,0 150,-150 z M-25,25 v-150 a150,150 0 0,0 -150,150 z", ro, jane, txt, arcsObj; g = new Cango(cvsID); g.setWorldCoordsRHC(xmin, ymin, xspan); gL1 = new Cango(g.createLayer()); gL1.dupCtx(g); jane = new Img("Images/JaneAvril2.jpg", { imgHeight: 100, border:true, lineWidthWC:6, strokeColor:'sienna', shadowOffsetX:8, shadowOffsetY:-8, shadowBlur:4, shadowColor:'grey' }); jane.scale(2); txt = new Text("Caption", { fillColor:"red", fontSize:20, border:true, lineWidth:1 }); arcsObj = new Shape(svgToCgo2D(Arc), { border:true, strokeColor:"red", lineWidth:2, // pixels fillColor:"yellow" }); arcsObj.scale(0.5); function drawBits() { jane.transform.translate(100, 250); g.render(jane, true); txt.transform.translate(100, 20); g.render(txt); gL1.render(arcsObj, true); } drawBits(); initZoomPan(g.createLayer(), [g, gL1], drawBits); }
shapeDefs
To simplify defining the most common shapes Cango provides the shapeDefs object which has methods to create the Cgo2D data arrays representing the outline path of many commonly used shapes. The methods take dimension parameters such as the width and height of the rectangle or the diameter of the circle.
Syntax:
var shapeData = shapeDefs.circle(d);
Description:
Generates a Cgo2D format data array defining the outline path of a circle with diameter 'd'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the circle.
Parameters:
d:Number - Diameter of the circle measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the circle.
Syntax:
var shapeData = shapeDefs.ellipse(w, h);
Description:
Generates a Cgo2D format data array defining the outline path of a ellipse with width (X axis) 'w' and height (Y axis) 'h'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the ellipse.
Parameters:
w:Number - The width (X dimension) of the ellipse measured in the world coordinates.
h:Number - The height (Y dimension) of the ellipse measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the ellipse.
Syntax:
var shapeData = shapeDefs.square(s);
Description:
Generates a Cgo2D format data array defining the outline path of a square with sides of length 's'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the square.
Parameters:
s:Number - Side length of the square measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the square.
Syntax:
var shapeData = shapeDefs.rectangle(w, h [, rad]);
Description:
Generates a Cgo2D format data array defining the outline path of a rectangle with width 'w' and height 'h'. The rectangle may optionally have its corners rounded with a user defined radius. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the rectangle.
Parameters:
w:Number - The width (X dimension) of the rectangle, measured in the world coordinates.
h:Number - The height (Y dimension) of the rectangle, measured in the world coordinates.
rad:Number - The radius of rounded corners applied to the rectangle, measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the rectangle.
Syntax:
var shapeData = shapeDefs.triangle(s);
Description:
Generates a Cgo2D format data array defining the outline path of an equilateral triangle with sides of length 's'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the triangle.
Parameters:
s:Number - The length of each side of the triangle measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the triangle.
Syntax:
var pathData = shapeDefs.cross(s);
Description:
Generates a Cgo2D format data array defining the outline path of a cross with horizontal and vertical arms of length 's'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the cross.
Parameters:
s:Number - The length of the horizontal and vertical arms of the cross, measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the cross.
Syntax:
var pathData = shapeDefs.ex(s);
Description:
Generates a Cgo2D format data array defining the outline path of a ex with arm lengths 's'. The returned array is suitable as input parameter for the Path, Shape and ClipMask objects. The drawing origin will be at the center of the ex.
Parameters:
s:Number - The length of each arm of the ex, measured in the world coordinates.
Returns:
Array - Array of Cgo2D format commands and associated coordinate pairs defining the outline of the ex.